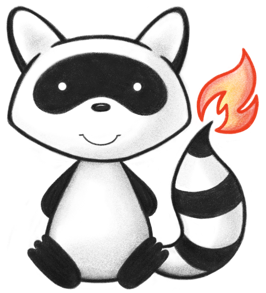
001package org.hl7.fhir.convertors.misc; 002 003 004import java.io.File; 005import java.io.FileInputStream; 006import java.io.FileNotFoundException; 007import java.io.FileOutputStream; 008import java.io.IOException; 009import java.sql.Connection; 010import java.sql.DriverManager; 011import java.sql.SQLException; 012import java.sql.Statement; 013import java.util.Date; 014import java.util.List; 015import java.util.ArrayList; 016import java.util.Scanner; 017 018import org.hl7.fhir.exceptions.FHIRException; 019import org.hl7.fhir.r5.formats.IParser.OutputStyle; 020import org.hl7.fhir.r5.formats.JsonParser; 021import org.hl7.fhir.r5.model.CodeSystem; 022import org.hl7.fhir.r5.model.BooleanType; 023import org.hl7.fhir.r5.model.StringType; 024import org.hl7.fhir.r5.model.CodeType; 025import org.hl7.fhir.r5.model.CodeSystem.ConceptDefinitionComponent; 026import org.hl7.fhir.r5.model.CodeSystem.ConceptDefinitionDesignationComponent; 027import org.hl7.fhir.r5.model.CodeSystem.ConceptPropertyComponent; 028import org.hl7.fhir.r5.model.CodeSystem.PropertyType; 029import org.hl7.fhir.r5.model.Coding; 030import org.hl7.fhir.r5.model.Enumerations.CodeSystemContentMode; 031import org.hl7.fhir.r5.model.Enumerations.PublicationStatus; 032import org.hl7.fhir.r5.terminologies.CodeSystemUtilities; 033import org.hl7.fhir.utilities.CSVReader; 034import org.hl7.fhir.utilities.Utilities; 035 036public class CPTImporter { 037 038 public static void main(String[] args) throws FHIRException, FileNotFoundException, IOException, ClassNotFoundException, SQLException { 039 new CPTImporter().doImport(args[0], args[1], args[2]); 040 041 } 042 043 044 private void doImport(String src, String version, String dst) throws FHIRException, FileNotFoundException, IOException, ClassNotFoundException, SQLException { 045 046 CodeSystem cs = new CodeSystem(); 047 cs.setId("cpt"); 048 cs.setUrl("http://www.ama-assn.org/go/cpt"); 049 cs.setVersion(version); 050 cs.setName("AmaCPT"); 051 cs.setTitle("AMA CPT"); 052 cs.setStatus(PublicationStatus.ACTIVE); 053 cs.setDate(new Date()); 054 cs.setContent(CodeSystemContentMode.COMPLETE); 055 cs.setCompositional(true); 056 cs.setPublisher("AMA"); 057 cs.setValueSet("http://hl7.org/fhir/ValueSet/cpt-all"); 058 cs.setCopyright("CPT © Copyright 2019 American Medical Association. All rights reserved. AMA and CPT are registered trademarks of the American Medical Association."); 059 cs.addProperty().setCode("modifier").setDescription("Whether code is a modifier code").setType(PropertyType.BOOLEAN); 060 cs.addProperty().setCode("modified").setDescription("Whether code has been modified (all base codes are not modified)").setType(PropertyType.BOOLEAN); 061 cs.addProperty().setCode("orthopox").setDescription("Whether code is one of the Pathology and Laboratory and Immunization Code(s) for Orthopoxvirus").setType(PropertyType.BOOLEAN); 062 cs.addProperty().setCode("telemedicine").setDescription("Whether code is appropriate for use with telemedicine (and the telemedicine modifier)").setType(PropertyType.BOOLEAN); 063 cs.addProperty().setCode("kind").setDescription("Kind of Code (see metadata)").setType(PropertyType.CODE); 064 065 defineMetadata(cs); 066 067 System.out.println("LONGULT: "+readCodes(cs, Utilities.path(src, "LONGULT.txt"), false, null, null, null)); 068 System.out.println("LONGUT: "+readCodes(cs, Utilities.path(src, "LONGUT.txt"), false, "upper", null, null)); 069 System.out.println("MEDU: "+readCodes(cs, Utilities.path(src, "MEDU.txt"), false, "med", null, null)); 070 System.out.println("SHORTU: "+readCodes(cs, Utilities.path(src, "SHORTU.txt"), false, "short", null, null)); 071 System.out.println("ConsumerDescriptor: "+readCodes(cs, Utilities.path(src, "ConsumerDescriptor.txt"), true, "consumer", null, null)); 072 System.out.println("ClinicianDescriptor: "+readCodes(cs, Utilities.path(src, "ClinicianDescriptor.txt"), true, "clinician", null, null)); 073 System.out.println("OrthopoxvirusCodes: "+readCodes(cs, Utilities.path(src, "OrthopoxvirusCodes.txt"), false, null, null, "orthopox")); 074 075 System.out.println("modifiers: "+processModifiers(cs, Utilities.path(src, "modifiers.csv"))); 076 System.out.println("appendix P: "+processAppendixP(cs)); 077 078 079 System.out.println("-------------------"); 080 System.out.println(cs.getConcept().size()); 081 int c = 0; 082 int k = 0; 083 for (ConceptDefinitionComponent cc: cs.getConcept()) { 084 c = Integer.max(c, cc.getProperty().size()); 085 if (cc.getProperty().size() > 3) { 086 k++; 087 } 088 } 089 System.out.println(c); 090 System.out.println(k); 091 092 new JsonParser().setOutputStyle(OutputStyle.PRETTY).compose(new FileOutputStream(dst), cs); 093 produceDB(Utilities.changeFileExt(dst, ".db"), cs); 094 095 cs.setContent(CodeSystemContentMode.FRAGMENT); 096 cs.getConcept().removeIf(cc -> !Utilities.existsInList(cc.getCode(), "metadata-kinds", "metadata-designations", "99202", "99203", "0001A", "99252", "25", "P1", "1P", "F1", "95")); 097 new JsonParser().setOutputStyle(OutputStyle.PRETTY).compose(new FileOutputStream(Utilities.changeFileExt(dst, "-fragment.json")), cs); 098 produceDB(Utilities.changeFileExt(dst, "-fragment.db"), cs); 099 } 100 101 private String processAppendixP(CodeSystem cs) { 102 List<String> tcodes = new ArrayList<>(); 103 tcodes.add("90785"); 104 tcodes.add("90791"); 105 tcodes.add("90792"); 106 tcodes.add("90832"); 107 tcodes.add("90833"); 108 tcodes.add("90834"); 109 tcodes.add("90836"); 110 tcodes.add("90837"); 111 tcodes.add("90838"); 112 tcodes.add("90839"); 113 tcodes.add("90840"); 114 tcodes.add("90845"); 115 tcodes.add("90846"); 116 tcodes.add("90847"); 117 tcodes.add("92507"); 118 tcodes.add("92508"); 119 tcodes.add("92521"); 120 tcodes.add("92522"); 121 tcodes.add("92523"); 122 tcodes.add("92524"); 123 tcodes.add("96040"); 124 tcodes.add("96110"); 125 tcodes.add("96116"); 126 tcodes.add("96160"); 127 tcodes.add("96161"); 128 tcodes.add("97802"); 129 tcodes.add("97803"); 130 tcodes.add("97804"); 131 tcodes.add("99406"); 132 tcodes.add("99407"); 133 tcodes.add("99408"); 134 tcodes.add("99409"); 135 tcodes.add("99497"); 136 tcodes.add("99498"); 137 138 for (String c : tcodes) { 139 ConceptDefinitionComponent cc = CodeSystemUtilities.findCode(cs.getConcept(), c); 140 if (cc == null) { 141 throw new Error("unable to find tcode "+c); 142 } 143 cc.addProperty().setCode("telemedicine").setValue(new BooleanType(true)); 144 } 145 return String.valueOf(tcodes.size()); 146 } 147 148 149 private void produceDB(String path, CodeSystem cs) throws ClassNotFoundException, SQLException { 150 Connection con = connect(path); 151 152 Statement stmt = con.createStatement(); 153 stmt.execute("insert into Information (name, value) values ('version', "+cs.getVersion()+")"); 154 for (ConceptDefinitionComponent cc: cs.getConcept()) { 155 if (!cc.getCode().startsWith("metadata")) { 156 stmt.execute("insert into Concepts (code, modifier) values ('"+cc.getCode()+"', "+isModifier(cc)+")"); 157 int i = 0; 158 if (cc.hasDisplay()) { 159 stmt.execute("insert into Designations (code, type, sequence, value) values ('"+cc.getCode()+"', 'display', 0, '"+Utilities.escapeSql(cc.getDisplay())+"')"); 160 i++; 161 } 162 for (ConceptDefinitionDesignationComponent d : cc.getDesignation()) { 163 stmt.execute("insert into Designations (code, type, sequence, value) values ('"+cc.getCode()+"', '"+d.getUse().getCode()+"', "+i+", '"+Utilities.escapeSql(d.getValue())+"')"); 164 i++; 165 } 166 i = 0; 167 for (ConceptPropertyComponent p : cc.getProperty()) { 168 if (!Utilities.existsInList(p.getCode(), "modified", "modifier")) { 169 stmt.execute("insert into Properties (code, name, sequence, value) values ('"+cc.getCode()+"', '"+p.getCode()+"', "+i+", '"+p.getValue().primitiveValue()+"')"); 170 i++; 171 } 172 } 173 } 174 } 175 176 } 177 178 private String isModifier(ConceptDefinitionComponent cc) { 179 for (ConceptPropertyComponent p : cc.getProperty()) { 180 if (p.getCode().equals("modifier")) { 181 return p.getValue().primitiveValue().equals("true") ? "1" : "0"; 182 } 183 } 184 return "0"; 185 } 186 187 188 private Connection connect(String dest) throws SQLException, ClassNotFoundException { 189 // Class.forName("com.mysql.jdbc.Driver"); 190 // con = DriverManager.getConnection("jdbc:mysql://localhost:3306/omop?useSSL=false","root",{pwd}); 191 new File(dest).delete(); 192 Connection con = DriverManager.getConnection("jdbc:sqlite:"+dest); 193 makeMetadataTable(con); 194 makeConceptsTable(con); 195 makeDesignationsTable(con); 196 makePropertiesTable(con); 197 return con; 198 } 199 200 private void makeDesignationsTable(Connection con) throws SQLException { 201 Statement stmt = con.createStatement(); 202 stmt.execute("CREATE TABLE Designations (\r\n"+ 203 "`code` varchar(15) NOT NULL,\r\n"+ 204 "`type` varchar(15) NOT NULL,\r\n"+ 205 "`sequence` int NOT NULL,\r\n"+ 206 "`value` text NOT NULL,\r\n"+ 207 "PRIMARY KEY (`code`, `type`, `sequence`))\r\n"); 208 } 209 210 211 private void makePropertiesTable(Connection con) throws SQLException { 212 213 Statement stmt = con.createStatement(); 214 stmt.execute("CREATE TABLE Properties (\r\n"+ 215 "`code` varchar(15) NOT NULL,\r\n"+ 216 "`name` varchar(15) NOT NULL,\r\n"+ 217 "`sequence` int NOT NULL,\r\n"+ 218 "`value` varchar(15) NOT NULL,\r\n"+ 219 "PRIMARY KEY (`code`, `name`, `sequence`))\r\n"); 220 221 } 222 223 224 private void makeConceptsTable(Connection con) throws SQLException { 225 226 Statement stmt = con.createStatement(); 227 stmt.execute("CREATE TABLE Concepts (\r\n"+ 228 "`code` varchar(15) NOT NULL,\r\n"+ 229 "`modifier` int DEFAULT NULL,\r\n"+ 230 "PRIMARY KEY (`code`))\r\n"); 231 232 } 233 234 235 private void makeMetadataTable(Connection con) throws SQLException { 236 237 Statement stmt = con.createStatement(); 238 stmt.execute("CREATE TABLE Information (\r\n"+ 239 "`name` varchar(64) NOT NULL,\r\n"+ 240 "`value` varchar(64) DEFAULT NULL,\r\n"+ 241 "PRIMARY KEY (`name`))\r\n"); 242 243 } 244 245 246 private void defineMetadata(CodeSystem cs) { 247 ConceptDefinitionComponent pc = mm(cs.addConcept().setCode("metadata-kinds")); 248 mm(pc.addConcept()).setCode("code").setDisplay("A normal CPT code"); 249 mm(pc.addConcept()).setCode("cat-1").setDisplay("CPT Level I Modifiers"); 250 mm(pc.addConcept()).setCode("cat-2").setDisplay("A Category II code or modifier"); 251 mm(pc.addConcept()).setCode("physical-status").setDisplay("Anesthesia Physical Status Modifiers"); 252 mm(pc.addConcept()).setCode("general").setDisplay("A general modifier"); 253 mm(pc.addConcept()).setCode("hcpcs").setDisplay("Level II (HCPCS/National) Modifiers"); 254 mm(pc.addConcept()).setCode("metadata").setDisplay("A kind of code or designation"); 255 256 ConceptDefinitionComponent dc = mm(cs.addConcept().setCode("metadata-designations")); 257 mm(dc.addConcept()).setCode("upper").setDisplay("Uppercase variant of the display"); 258 mm(dc.addConcept()).setCode("med").setDisplay("Medium length variant of the display (all uppercase)"); 259 mm(dc.addConcept()).setCode("short").setDisplay("Short length variant of the display (all uppercase)"); 260 mm(dc.addConcept()).setCode("consumer").setDisplay("Consumer Friendly representation for the concept"); 261 mm(dc.addConcept()).setCode("clinician").setDisplay("Clinician Friendly representation for the concept (can be more than one per concept)"); 262 } 263 264 private ConceptDefinitionComponent mm(ConceptDefinitionComponent cc) { 265 cc.addProperty().setCode("kind").setValue(new CodeType("metadata")); 266 return cc; 267 } 268 269 private int processModifiers(CodeSystem cs, String path) throws FHIRException, FileNotFoundException, IOException { 270 CSVReader csv = new CSVReader(new FileInputStream(path)); 271 csv.readHeaders(); 272 273 int res = 0; 274 while (csv.line()) { 275 String code = csv.cell("Code"); 276 String general = csv.cell("General"); 277 String physicalStatus = csv.cell("PhysicalStatus"); 278 String levelOne = csv.cell("LevelOne"); 279 String levelTwo = csv.cell("LevelTwo"); 280 String hcpcs = csv.cell("HCPCS"); 281 String defn = csv.cell("Definition"); 282 283 res = Integer.max(res, defn.length()); 284 ConceptDefinitionComponent cc = cs.addConcept().setCode(code); 285 cc.setDisplay(defn); 286 cc.addProperty().setCode("modified").setValue(new BooleanType(false)); 287 cc.addProperty().setCode("modifier").setValue(new BooleanType(true)); 288 if ("1".equals(general)) { 289 cc.addProperty().setCode("kind").setValue(new CodeType("general")); 290 } 291 if ("1".equals(physicalStatus)) { 292 cc.addProperty().setCode("kind").setValue(new CodeType("physical-status")); 293 } 294 if ("1".equals(levelOne)) { 295 cc.addProperty().setCode("kind").setValue(new CodeType("cat-1")); 296 } 297 if ("1".equals(levelTwo)) { 298 cc.addProperty().setCode("kind").setValue(new CodeType("cat-2")); 299 } 300 if ("1".equals(hcpcs)) { 301 cc.addProperty().setCode("kind").setValue(new CodeType("hcpcs")); 302 } 303 } 304 return res; 305 } 306 307 private int readCodes(CodeSystem cs, String path, boolean hasConceptId, String use, String type, String boolProp) throws IOException { 308 int res = 0; 309 FileInputStream inputStream = null; 310 Scanner sc = null; 311 try { 312 inputStream = new FileInputStream(path); 313 sc = new Scanner(inputStream, "UTF-8"); 314 while (sc.hasNextLine()) { 315 String line = sc.nextLine(); 316 if (hasConceptId) { 317 line = line.substring(7).trim(); 318 } 319 String code = line.substring(0, 5); 320 String desc = line.substring(6); 321 if (desc.contains("\t")) { 322 desc = desc.substring(desc.indexOf("\t")+1); 323 } 324 res = Integer.max(res, desc.length()); 325 ConceptDefinitionComponent cc = CodeSystemUtilities.getCode(cs, code); 326 if (cc == null) { 327 cc = cs.addConcept().setCode(code); 328 cc.addProperty().setCode("modifier").setValue(new BooleanType(false)); 329 cc.addProperty().setCode("modified").setValue(new BooleanType(false)); 330 if (type == null) { 331 if (Utilities.isInteger(code)) { 332 cc.addProperty().setCode("kind").setValue(new CodeType("code")); 333 } else { 334 cc.addProperty().setCode("kind").setValue(new CodeType("cat-2")); 335 } 336 } else { 337 cc.addProperty().setCode("kind").setValue(new CodeType(type)); 338 } 339 } else if (type != null) { 340 cc.addProperty().setCode("kind").setValue(new CodeType(type)); 341 } 342 if (boolProp != null) { 343 cc.addProperty().setCode(boolProp).setValue(new BooleanType(true)); 344 } 345 if (use == null) { 346 if (cc.hasDisplay()) { 347 System.err.println("?"); 348 } 349 cc.setDisplay(desc); 350 } else { 351 cc.addDesignation().setUse(new Coding("http://www.ama-assn.org/go/cpt", use, null)).setValue(desc); 352 } 353 } 354 // note that Scanner suppresses exceptions 355 if (sc.ioException() != null) { 356 throw sc.ioException(); 357 } 358 } finally { 359 if (inputStream != null) { 360 inputStream.close(); 361 } 362 if (sc != null) { 363 sc.close(); 364 } 365 } 366 return res; 367 } 368 369 370}