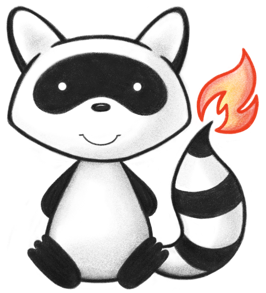
001package org.hl7.fhir.convertors.misc; 002 003import java.io.ByteArrayInputStream; 004import java.io.File; 005import java.io.InputStream; 006import java.util.ArrayList; 007import java.util.List; 008 009import javax.xml.parsers.DocumentBuilder; 010import javax.xml.parsers.DocumentBuilderFactory; 011 012/* 013 Copyright (c) 2011+, HL7, Inc. 014 All rights reserved. 015 016 Redistribution and use in source and binary forms, with or without modification, 017 are permitted provided that the following conditions are met: 018 019 * Redistributions of source code must retain the above copyright notice, this 020 list of conditions and the following disclaimer. 021 * Redistributions in binary form must reproduce the above copyright notice, 022 this list of conditions and the following disclaimer in the documentation 023 and/or other materials provided with the distribution. 024 * Neither the name of HL7 nor the names of its contributors may be used to 025 endorse or promote products derived from this software without specific 026 prior written permission. 027 028 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 029 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 030 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 031 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 032 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 033 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 034 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 035 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 036 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 037 POSSIBILITY OF SUCH DAMAGE. 038 039 */ 040 041 042import org.hl7.fhir.convertors.misc.adl.ADLImporter; 043import org.hl7.fhir.utilities.SimpleHTTPClient; 044import org.hl7.fhir.utilities.SimpleHTTPClient.HTTPResult; 045import org.hl7.fhir.utilities.TextFile; 046import org.hl7.fhir.utilities.Utilities; 047import org.hl7.fhir.utilities.xml.XMLUtil; 048import org.w3c.dom.Document; 049import org.w3c.dom.Element; 050 051public class CKMImporter { 052 053 private String ckm; 054 private String dest; 055 private String config; 056 private String info; 057 058 public static void main(String[] args) throws Exception { 059 CKMImporter self = new CKMImporter(); 060 self.ckm = getParam(args, "ckm"); 061 self.dest = getParam(args, "dest"); 062 self.config = getParam(args, "config"); 063 self.info = getParam(args, "info"); 064 if (self.ckm == null || self.dest == null || self.config == null) { 065 System.out.println("ADL to FHIR StructureDefinition Converter"); 066 System.out.println("This tool takes 4 parameters:"); 067 System.out.println("-ckm: Baase URL of CKM"); 068 System.out.println("-dest: folder for output"); 069 System.out.println("-config: filename of OpenEHR/FHIR knowlege base (required)"); 070 System.out.println("-info: folder for additional knowlege of archetypes"); 071 } else { 072 self.execute(); 073 } 074 } 075 076 private static String getParam(String[] args, String name) { 077 for (int i = 0; i < args.length - 1; i++) { 078 if (args[i].equals("-" + name)) { 079 return args[i + 1]; 080 } 081 } 082 return null; 083 } 084 085 086 private void execute() throws Exception { 087 List<String> ids = new ArrayList<String>(); 088 Document xml = loadXml(ckm + "/services/ArchetypeFinderBean/getAllArchetypeIds"); 089 Element e = XMLUtil.getFirstChild(xml.getDocumentElement()); 090 while (e != null) { 091 ids.add(e.getTextContent()); 092 e = XMLUtil.getNextSibling(e); 093 } 094// for (String id : ids) { 095// downloadArchetype(id); 096// } 097 for (String id : ids) { 098 processArchetype(id); 099 } 100 } 101 102 private void downloadArchetype(String id) throws Exception { 103 System.out.println("Fetch " + id); 104 Document sxml = loadXml(ckm + "/services/ArchetypeFinderBean/getArchetypeInXML?archetypeId=" + id); 105 Element e = XMLUtil.getFirstChild(sxml.getDocumentElement()); 106 107 String src = Utilities.path(Utilities.path("[tmp]"), id + ".xml"); 108 TextFile.stringToFile(e.getTextContent(), src); 109 } 110 111 private void processArchetype(String id) throws Exception { 112 System.out.println("Process " + id); 113 114 String cfg = info == null ? null : Utilities.path(info, id + ".config"); 115 String src = Utilities.path(Utilities.path("[tmp]"), id + ".xml"); 116 String dst = Utilities.path(dest, id + ".xml"); 117 118 if (!new File(src).exists()) 119 downloadArchetype(id); 120 if (cfg != null && new File(cfg).exists()) 121 ADLImporter.main(new String[]{"-source", src, "-dest", dst, "-config", config, "-info", cfg}); 122 else 123 ADLImporter.main(new String[]{"-source", src, "-dest", dst, "-config", config}); 124 } 125 126 private Document loadXml(String address) throws Exception { 127 SimpleHTTPClient http = new SimpleHTTPClient(); 128 HTTPResult res = http.get(address, "application/xml"); 129 res.checkThrowException(); 130 InputStream xml = new ByteArrayInputStream(res.getContent()); 131 132 DocumentBuilderFactory dbf = DocumentBuilderFactory.newInstance(); 133 DocumentBuilder db = dbf.newDocumentBuilder(); 134 return db.parse(xml); 135 } 136}