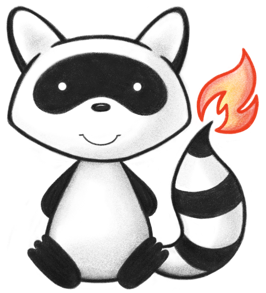
001package org.hl7.fhir.convertors.conv43_50.resources43_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext43_50; 004import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.CodeableConcept43_50; 005import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Canonical43_50; 006import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Instant43_50; 007import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Integer64_43_50; 008import org.hl7.fhir.convertors.conv43_50.datatypes43_50.special43_50.Reference43_50; 009 010public class SubscriptionStatus43_50 { 011 public static org.hl7.fhir.r4b.model.SubscriptionStatus convertSubscriptionStatus(org.hl7.fhir.r5.model.SubscriptionStatus src) { 012 if (src == null) 013 return null; 014 org.hl7.fhir.r4b.model.SubscriptionStatus tgt = new org.hl7.fhir.r4b.model.SubscriptionStatus(); 015 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyResource(src, tgt); 016 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyDomainResource(src, tgt); 017 018 if (src.hasType()) 019 { 020 tgt.setTypeElement(convertSubscriptionNotificationType(src.getTypeElement())); 021 } 022 if (src.hasStatus()) { 023 tgt.setStatusElement(convertSubscriptionStatus(src.getStatusElement())); 024 } 025 if (src.hasEventsSinceSubscriptionStart()) { 026 org.hl7.fhir.r4b.model.StringType value = new org.hl7.fhir.r4b.model.StringType(); 027 value.setValue(src.getEventsSinceSubscriptionStartElement().getValueAsString()); 028 tgt.setEventsSinceSubscriptionStartElement(value); 029 } 030 if (src.hasNotificationEvent()) { 031 for (org.hl7.fhir.r5.model.SubscriptionStatus.SubscriptionStatusNotificationEventComponent element : src.getNotificationEvent()) { 032 tgt.addNotificationEvent(convertSubscriptionStatusNotificationEventComponent(element)); 033 } 034 } 035 if (src.hasSubscription()) { 036 tgt.setSubscription(Reference43_50.convertReference(src.getSubscription())); 037 } 038 if (src.hasTopic()) { 039 tgt.setTopicElement(Canonical43_50.convertCanonical(src.getTopicElement())); 040 } 041 if (src.hasError()) { 042 for (org.hl7.fhir.r5.model.CodeableConcept srcError : src.getError()) { 043 tgt.addError(CodeableConcept43_50.convertCodeableConcept(srcError)); 044 } 045 } 046 return tgt; 047 } 048 049 private static org.hl7.fhir.r4b.model.SubscriptionStatus.SubscriptionStatusNotificationEventComponent convertSubscriptionStatusNotificationEventComponent(org.hl7.fhir.r5.model.SubscriptionStatus.SubscriptionStatusNotificationEventComponent src) { 050 org.hl7.fhir.r4b.model.SubscriptionStatus.SubscriptionStatusNotificationEventComponent tgt = new org.hl7.fhir.r4b.model.SubscriptionStatus.SubscriptionStatusNotificationEventComponent(); 051 if (src.hasEventNumber()) { 052 tgt.setEventNumberElement(Integer64_43_50.convertInteger64ToString(src.getEventNumberElement())); 053 } 054 if (src.hasTimestamp()) { 055 tgt.setTimestampElement(Instant43_50.convertInstant(src.getTimestampElement())); 056 } 057 if (src.hasFocus()) { 058 tgt.setFocus(Reference43_50.convertReference(src.getFocus())); 059 } 060 if (src.hasAdditionalContext()) { 061 for (org.hl7.fhir.r5.model.Reference ref : src.getAdditionalContext()) { 062 tgt.addAdditionalContext(Reference43_50.convertReference(ref)); 063 } 064 } 065 return tgt; 066 } 067 068 private static org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.SubscriptionStatus> convertSubscriptionStatus(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.SubscriptionStatusCodes> src) { 069 org.hl7.fhir.r4b.model.Enumerations.SubscriptionStatusEnumFactory enumFactory = new org.hl7.fhir.r4b.model.Enumerations.SubscriptionStatusEnumFactory(); 070 switch(src.getValue()) { 071 case ACTIVE: return new org.hl7.fhir.r4b.model.Enumeration<>(enumFactory, org.hl7.fhir.r4b.model.Enumerations.SubscriptionStatus.ACTIVE); 072 case ERROR: return new org.hl7.fhir.r4b.model.Enumeration<>(enumFactory, org.hl7.fhir.r4b.model.Enumerations.SubscriptionStatus.ERROR); 073 case ENTEREDINERROR: return new org.hl7.fhir.r4b.model.Enumeration<>(enumFactory, org.hl7.fhir.r4b.model.Enumerations.SubscriptionStatus.ERROR); 074 case OFF: return new org.hl7.fhir.r4b.model.Enumeration<>(enumFactory, org.hl7.fhir.r4b.model.Enumerations.SubscriptionStatus.OFF); 075 case REQUESTED: return new org.hl7.fhir.r4b.model.Enumeration<>(enumFactory, org.hl7.fhir.r4b.model.Enumerations.SubscriptionStatus.REQUESTED); 076 case NULL: return new org.hl7.fhir.r4b.model.Enumeration<>(enumFactory, org.hl7.fhir.r4b.model.Enumerations.SubscriptionStatus.NULL); 077 } 078 return null; 079 } 080 081 private static org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.SubscriptionStatus.SubscriptionNotificationType> convertSubscriptionNotificationType(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.SubscriptionStatus.SubscriptionNotificationType> src) { 082 org.hl7.fhir.r4b.model.SubscriptionStatus.SubscriptionNotificationTypeEnumFactory enumFactory = new org.hl7.fhir.r4b.model.SubscriptionStatus.SubscriptionNotificationTypeEnumFactory(); 083 switch(src.getValue()) { 084 case EVENTNOTIFICATION: return new org.hl7.fhir.r4b.model.Enumeration<>(enumFactory, org.hl7.fhir.r4b.model.SubscriptionStatus.SubscriptionNotificationType.EVENTNOTIFICATION); 085 case HANDSHAKE: return new org.hl7.fhir.r4b.model.Enumeration<>(enumFactory, org.hl7.fhir.r4b.model.SubscriptionStatus.SubscriptionNotificationType.HANDSHAKE); 086 case HEARTBEAT: return new org.hl7.fhir.r4b.model.Enumeration<>(enumFactory, org.hl7.fhir.r4b.model.SubscriptionStatus.SubscriptionNotificationType.HEARTBEAT); 087 case QUERYEVENT: return new org.hl7.fhir.r4b.model.Enumeration<>(enumFactory, org.hl7.fhir.r4b.model.SubscriptionStatus.SubscriptionNotificationType.QUERYEVENT); 088 case QUERYSTATUS: return new org.hl7.fhir.r4b.model.Enumeration<>(enumFactory, org.hl7.fhir.r4b.model.SubscriptionStatus.SubscriptionNotificationType.QUERYSTATUS); 089 case NULL: return new org.hl7.fhir.r4b.model.Enumeration<>(enumFactory, org.hl7.fhir.r4b.model.SubscriptionStatus.SubscriptionNotificationType.NULL); 090 } 091 return null; 092 } 093 094 095 public static org.hl7.fhir.r5.model.SubscriptionStatus convertSubscriptionStatus(org.hl7.fhir.r4b.model.SubscriptionStatus src) { 096 if (src == null) 097 return null; 098 org.hl7.fhir.r5.model.SubscriptionStatus tgt = new org.hl7.fhir.r5.model.SubscriptionStatus(); 099 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyResource(src, tgt); 100 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyDomainResource(src, tgt); 101 102 if (src.hasType()) 103 { 104 tgt.setTypeElement(convertSubscriptionNotificationType(src.getTypeElement())); 105 } 106 if (src.hasStatus()) { 107 tgt.setStatusElement(convertSubscriptionStatus(src.getStatusElement())); 108 } 109 if (src.hasEventsSinceSubscriptionStart()) { 110 org.hl7.fhir.r5.model.Integer64Type value = new org.hl7.fhir.r5.model.Integer64Type(); 111 value.fromStringValue(src.getEventsSinceSubscriptionStartElement().getValueAsString()); 112 tgt.setEventsSinceSubscriptionStartElement(value); 113 } 114 if (src.hasNotificationEvent()) { 115 for (org.hl7.fhir.r4b.model.SubscriptionStatus.SubscriptionStatusNotificationEventComponent element : src.getNotificationEvent()) { 116 tgt.addNotificationEvent(convertSubscriptionStatusNotificationEventComponent(element)); 117 } 118 } 119 if (src.hasSubscription()) { 120 tgt.setSubscription(Reference43_50.convertReference(src.getSubscription())); 121 } 122 if (src.hasTopic()) { 123 tgt.setTopicElement(Canonical43_50.convertCanonical(src.getTopicElement())); 124 } 125 if (src.hasError()) { 126 for (org.hl7.fhir.r4b.model.CodeableConcept srcError : src.getError()) { 127 tgt.addError(CodeableConcept43_50.convertCodeableConcept(srcError)); 128 } 129 } 130 return tgt; 131 } 132 133 private static org.hl7.fhir.r5.model.SubscriptionStatus.SubscriptionStatusNotificationEventComponent convertSubscriptionStatusNotificationEventComponent(org.hl7.fhir.r4b.model.SubscriptionStatus.SubscriptionStatusNotificationEventComponent src) { 134 org.hl7.fhir.r5.model.SubscriptionStatus.SubscriptionStatusNotificationEventComponent tgt = new org.hl7.fhir.r5.model.SubscriptionStatus.SubscriptionStatusNotificationEventComponent(); 135 if (src.hasEventNumber()) { 136 tgt.setEventNumberElement(Integer64_43_50.convertStringToInteger64(src.getEventNumberElement())); 137 } 138 if (src.hasTimestamp()) { 139 tgt.setTimestampElement(Instant43_50.convertInstant(src.getTimestampElement())); 140 } 141 if (src.hasFocus()) { 142 tgt.setFocus(Reference43_50.convertReference(src.getFocus())); 143 } 144 if (src.hasAdditionalContext()) { 145 for (org.hl7.fhir.r4b.model.Reference ref : src.getAdditionalContext()) { 146 tgt.addAdditionalContext(Reference43_50.convertReference(ref)); 147 } 148 } 149 return tgt; 150 } 151 152 private static org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.SubscriptionStatusCodes> convertSubscriptionStatus(org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.SubscriptionStatus> src) { 153 org.hl7.fhir.r5.model.Enumerations.SubscriptionStatusCodesEnumFactory enumFactory = new org.hl7.fhir.r5.model.Enumerations.SubscriptionStatusCodesEnumFactory(); 154 switch(src.getValue()) { 155 case ACTIVE: return new org.hl7.fhir.r5.model.Enumeration<>(enumFactory, org.hl7.fhir.r5.model.Enumerations.SubscriptionStatusCodes.ACTIVE); 156 case ERROR: return new org.hl7.fhir.r5.model.Enumeration<>(enumFactory, org.hl7.fhir.r5.model.Enumerations.SubscriptionStatusCodes.ERROR); 157 case OFF: return new org.hl7.fhir.r5.model.Enumeration<>(enumFactory, org.hl7.fhir.r5.model.Enumerations.SubscriptionStatusCodes.OFF); 158 case REQUESTED: return new org.hl7.fhir.r5.model.Enumeration<>(enumFactory, org.hl7.fhir.r5.model.Enumerations.SubscriptionStatusCodes.REQUESTED); 159 case NULL: return new org.hl7.fhir.r5.model.Enumeration<>(enumFactory, org.hl7.fhir.r5.model.Enumerations.SubscriptionStatusCodes.NULL); 160 } 161 return null; 162 } 163 164 private static org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.SubscriptionStatus.SubscriptionNotificationType> convertSubscriptionNotificationType(org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.SubscriptionStatus.SubscriptionNotificationType> src) { 165 org.hl7.fhir.r5.model.SubscriptionStatus.SubscriptionNotificationTypeEnumFactory enumFactory = new org.hl7.fhir.r5.model.SubscriptionStatus.SubscriptionNotificationTypeEnumFactory(); 166 switch(src.getValue()) { 167 case EVENTNOTIFICATION: return new org.hl7.fhir.r5.model.Enumeration<>(enumFactory, org.hl7.fhir.r5.model.SubscriptionStatus.SubscriptionNotificationType.EVENTNOTIFICATION); 168 case HANDSHAKE: return new org.hl7.fhir.r5.model.Enumeration<>(enumFactory, org.hl7.fhir.r5.model.SubscriptionStatus.SubscriptionNotificationType.HANDSHAKE); 169 case HEARTBEAT: return new org.hl7.fhir.r5.model.Enumeration<>(enumFactory, org.hl7.fhir.r5.model.SubscriptionStatus.SubscriptionNotificationType.HEARTBEAT); 170 case QUERYEVENT: return new org.hl7.fhir.r5.model.Enumeration<>(enumFactory, org.hl7.fhir.r5.model.SubscriptionStatus.SubscriptionNotificationType.QUERYEVENT); 171 case QUERYSTATUS: return new org.hl7.fhir.r5.model.Enumeration<>(enumFactory, org.hl7.fhir.r5.model.SubscriptionStatus.SubscriptionNotificationType.QUERYSTATUS); 172 case NULL: return new org.hl7.fhir.r5.model.Enumeration<>(enumFactory, org.hl7.fhir.r5.model.SubscriptionStatus.SubscriptionNotificationType.NULL); 173 } 174 return null; 175 } 176}