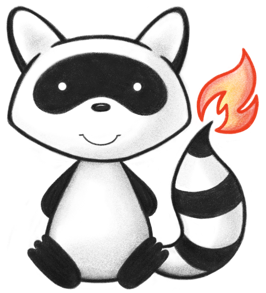
001package org.hl7.fhir.convertors.conv30_50.resources30_50; 002 003import java.util.stream.Collectors; 004 005import org.hl7.fhir.convertors.context.ConversionContext30_50; 006import org.hl7.fhir.convertors.conv30_50.datatypes30_50.Reference30_50; 007import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.Annotation30_50; 008import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.CodeableConcept30_50; 009import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.Identifier30_50; 010import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.DateTime30_50; 011import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.String30_50; 012import org.hl7.fhir.exceptions.FHIRException; 013import org.hl7.fhir.r5.model.AllergyIntolerance.AllergyIntoleranceParticipantComponent; 014import org.hl7.fhir.r5.model.CodeableConcept; 015import org.hl7.fhir.r5.model.CodeableReference; 016import org.hl7.fhir.r5.model.Coding; 017 018public class AllergyIntolerance30_50 { 019 020 public static org.hl7.fhir.dstu3.model.AllergyIntolerance convertAllergyIntolerance(org.hl7.fhir.r5.model.AllergyIntolerance src) throws FHIRException { 021 if (src == null) 022 return null; 023 org.hl7.fhir.dstu3.model.AllergyIntolerance tgt = new org.hl7.fhir.dstu3.model.AllergyIntolerance(); 024 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyDomainResource(src, tgt); 025 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 026 tgt.addIdentifier(Identifier30_50.convertIdentifier(t)); 027 if (src.hasClinicalStatus()) 028 tgt.setClinicalStatus(convertAllergyIntoleranceClinicalStatus(src.getClinicalStatus())); 029 if (src.hasVerificationStatus()) 030 tgt.setVerificationStatus(convertAllergyIntoleranceVerificationStatus(src.getVerificationStatus())); 031 if (src.hasType()) 032 tgt.setTypeElement(convertAllergyIntoleranceType(src.getType())); 033 tgt.setCategory(src.getCategory().stream() 034 .map(AllergyIntolerance30_50::convertAllergyIntoleranceCategory) 035 .collect(Collectors.toList())); 036 if (src.hasCriticality()) 037 tgt.setCriticalityElement(convertAllergyIntoleranceCriticality(src.getCriticalityElement())); 038 if (src.hasCode()) 039 tgt.setCode(CodeableConcept30_50.convertCodeableConcept(src.getCode())); 040 if (src.hasPatient()) 041 tgt.setPatient(Reference30_50.convertReference(src.getPatient())); 042 if (src.hasOnset()) 043 tgt.setOnset(ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().convertType(src.getOnset())); 044 if (src.hasRecordedDate()) 045 tgt.setAssertedDateElement(DateTime30_50.convertDateTime(src.getRecordedDateElement())); 046 for (AllergyIntoleranceParticipantComponent t : src.getParticipant()) { 047 if (t.getFunction().hasCoding("http://terminology.hl7.org/CodeSystem/provenance-participant-type", "author")) 048 tgt.setRecorder(Reference30_50.convertReference(t.getActor())); 049 if (t.getFunction().hasCoding("http://terminology.hl7.org/CodeSystem/provenance-participant-type", "attester")) 050 tgt.setAsserter(Reference30_50.convertReference(t.getActor())); 051 } 052 if (src.hasLastOccurrence()) 053 tgt.setLastOccurrenceElement(DateTime30_50.convertDateTime(src.getLastOccurrenceElement())); 054 for (org.hl7.fhir.r5.model.Annotation t : src.getNote()) tgt.addNote(Annotation30_50.convertAnnotation(t)); 055 for (org.hl7.fhir.r5.model.AllergyIntolerance.AllergyIntoleranceReactionComponent t : src.getReaction()) 056 tgt.addReaction(convertAllergyIntoleranceReactionComponent(t)); 057 return tgt; 058 } 059 060 public static org.hl7.fhir.r5.model.AllergyIntolerance convertAllergyIntolerance(org.hl7.fhir.dstu3.model.AllergyIntolerance src) throws FHIRException { 061 if (src == null) 062 return null; 063 org.hl7.fhir.r5.model.AllergyIntolerance tgt = new org.hl7.fhir.r5.model.AllergyIntolerance(); 064 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyDomainResource(src, tgt); 065 for (org.hl7.fhir.dstu3.model.Identifier t : src.getIdentifier()) 066 tgt.addIdentifier(Identifier30_50.convertIdentifier(t)); 067 if (src.hasClinicalStatus()) 068 tgt.setClinicalStatus(convertAllergyIntoleranceClinicalStatus(src.getClinicalStatus())); 069 if (src.hasVerificationStatus()) 070 tgt.setVerificationStatus(convertAllergyIntoleranceVerificationStatus(src.getVerificationStatus())); 071 if (src.hasType()) 072 tgt.setType(convertAllergyIntoleranceType(src.getTypeElement())); 073 tgt.setCategory(src.getCategory().stream() 074 .map(AllergyIntolerance30_50::convertAllergyIntoleranceCategory) 075 .collect(Collectors.toList())); 076 if (src.hasCriticality()) 077 tgt.setCriticalityElement(convertAllergyIntoleranceCriticality(src.getCriticalityElement())); 078 if (src.hasCode()) 079 tgt.setCode(CodeableConcept30_50.convertCodeableConcept(src.getCode())); 080 if (src.hasPatient()) 081 tgt.setPatient(Reference30_50.convertReference(src.getPatient())); 082 if (src.hasOnset()) 083 tgt.setOnset(ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().convertType(src.getOnset())); 084 if (src.hasAssertedDate()) 085 tgt.setRecordedDateElement(DateTime30_50.convertDateTime(src.getAssertedDateElement())); 086 if (src.hasRecorder()) 087 tgt.addParticipant(new AllergyIntoleranceParticipantComponent() 088 .setFunction(new CodeableConcept().addCoding(new Coding("http://terminology.hl7.org/CodeSystem/provenance-participant-type", "author", "Author"))) 089 .setActor(Reference30_50.convertReference(src.getRecorder()))); 090 if (src.hasAsserter()) 091 tgt.addParticipant(new AllergyIntoleranceParticipantComponent() 092 .setFunction(new CodeableConcept().addCoding(new Coding("http://terminology.hl7.org/CodeSystem/provenance-participant-type", "attester", "Attester"))) 093 .setActor(Reference30_50.convertReference(src.getRecorder()))); 094 if (src.hasLastOccurrence()) 095 tgt.setLastOccurrenceElement(DateTime30_50.convertDateTime(src.getLastOccurrenceElement())); 096 for (org.hl7.fhir.dstu3.model.Annotation t : src.getNote()) tgt.addNote(Annotation30_50.convertAnnotation(t)); 097 for (org.hl7.fhir.dstu3.model.AllergyIntolerance.AllergyIntoleranceReactionComponent t : src.getReaction()) 098 tgt.addReaction(convertAllergyIntoleranceReactionComponent(t)); 099 return tgt; 100 } 101 102 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.AllergyIntolerance.AllergyIntoleranceCategory> convertAllergyIntoleranceCategory(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.AllergyIntolerance.AllergyIntoleranceCategory> src) throws FHIRException { 103 if (src == null || src.isEmpty()) 104 return null; 105 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.AllergyIntolerance.AllergyIntoleranceCategory> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.AllergyIntolerance.AllergyIntoleranceCategoryEnumFactory()); 106 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 107 switch (src.getValue()) { 108 case FOOD: 109 tgt.setValue(org.hl7.fhir.r5.model.AllergyIntolerance.AllergyIntoleranceCategory.FOOD); 110 break; 111 case MEDICATION: 112 tgt.setValue(org.hl7.fhir.r5.model.AllergyIntolerance.AllergyIntoleranceCategory.MEDICATION); 113 break; 114 case ENVIRONMENT: 115 tgt.setValue(org.hl7.fhir.r5.model.AllergyIntolerance.AllergyIntoleranceCategory.ENVIRONMENT); 116 break; 117 case BIOLOGIC: 118 tgt.setValue(org.hl7.fhir.r5.model.AllergyIntolerance.AllergyIntoleranceCategory.BIOLOGIC); 119 break; 120 default: 121 tgt.setValue(org.hl7.fhir.r5.model.AllergyIntolerance.AllergyIntoleranceCategory.NULL); 122 break; 123 } 124 return tgt; 125 } 126 127 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.AllergyIntolerance.AllergyIntoleranceCategory> convertAllergyIntoleranceCategory(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.AllergyIntolerance.AllergyIntoleranceCategory> src) throws FHIRException { 128 if (src == null || src.isEmpty()) 129 return null; 130 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.AllergyIntolerance.AllergyIntoleranceCategory> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.AllergyIntolerance.AllergyIntoleranceCategoryEnumFactory()); 131 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 132 switch (src.getValue()) { 133 case FOOD: 134 tgt.setValue(org.hl7.fhir.dstu3.model.AllergyIntolerance.AllergyIntoleranceCategory.FOOD); 135 break; 136 case MEDICATION: 137 tgt.setValue(org.hl7.fhir.dstu3.model.AllergyIntolerance.AllergyIntoleranceCategory.MEDICATION); 138 break; 139 case ENVIRONMENT: 140 tgt.setValue(org.hl7.fhir.dstu3.model.AllergyIntolerance.AllergyIntoleranceCategory.ENVIRONMENT); 141 break; 142 case BIOLOGIC: 143 tgt.setValue(org.hl7.fhir.dstu3.model.AllergyIntolerance.AllergyIntoleranceCategory.BIOLOGIC); 144 break; 145 default: 146 tgt.setValue(org.hl7.fhir.dstu3.model.AllergyIntolerance.AllergyIntoleranceCategory.NULL); 147 break; 148 } 149 return tgt; 150 } 151 152 static public org.hl7.fhir.dstu3.model.AllergyIntolerance.AllergyIntoleranceClinicalStatus convertAllergyIntoleranceClinicalStatus(org.hl7.fhir.r5.model.CodeableConcept src) throws FHIRException { 153 if (src == null) 154 return null; 155 if (src.hasCoding("http://terminology.hl7.org/CodeSystem/allergyintolerance-clinical", "active")) 156 return org.hl7.fhir.dstu3.model.AllergyIntolerance.AllergyIntoleranceClinicalStatus.ACTIVE; 157 if (src.hasCoding("http://terminology.hl7.org/CodeSystem/allergyintolerance-clinical", "inactive")) 158 return org.hl7.fhir.dstu3.model.AllergyIntolerance.AllergyIntoleranceClinicalStatus.INACTIVE; 159 if (src.hasCoding("http://terminology.hl7.org/CodeSystem/allergyintolerance-clinical", "resolved")) 160 return org.hl7.fhir.dstu3.model.AllergyIntolerance.AllergyIntoleranceClinicalStatus.RESOLVED; 161 return org.hl7.fhir.dstu3.model.AllergyIntolerance.AllergyIntoleranceClinicalStatus.NULL; 162 } 163 164 static public org.hl7.fhir.r5.model.CodeableConcept convertAllergyIntoleranceClinicalStatus(org.hl7.fhir.dstu3.model.AllergyIntolerance.AllergyIntoleranceClinicalStatus src) throws FHIRException { 165 if (src == null) 166 return null; 167 switch (src) { 168 case ACTIVE: 169 return new org.hl7.fhir.r5.model.CodeableConcept(new org.hl7.fhir.r5.model.Coding().setSystem("http://terminology.hl7.org/CodeSystem/allergyintolerance-clinical").setCode("active")); 170 case INACTIVE: 171 return new org.hl7.fhir.r5.model.CodeableConcept(new org.hl7.fhir.r5.model.Coding().setSystem("http://terminology.hl7.org/CodeSystem/allergyintolerance-clinical").setCode("inactive")); 172 case RESOLVED: 173 new org.hl7.fhir.r5.model.CodeableConcept(new org.hl7.fhir.r5.model.Coding().setSystem("http://terminology.hl7.org/CodeSystem/allergyintolerance-clinical").setCode("resolved")); 174 default: 175 return null; 176 } 177 } 178 179 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.AllergyIntolerance.AllergyIntoleranceCriticality> convertAllergyIntoleranceCriticality(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.AllergyIntolerance.AllergyIntoleranceCriticality> src) throws FHIRException { 180 if (src == null || src.isEmpty()) 181 return null; 182 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.AllergyIntolerance.AllergyIntoleranceCriticality> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.AllergyIntolerance.AllergyIntoleranceCriticalityEnumFactory()); 183 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 184 switch (src.getValue()) { 185 case LOW: 186 tgt.setValue(org.hl7.fhir.dstu3.model.AllergyIntolerance.AllergyIntoleranceCriticality.LOW); 187 break; 188 case HIGH: 189 tgt.setValue(org.hl7.fhir.dstu3.model.AllergyIntolerance.AllergyIntoleranceCriticality.HIGH); 190 break; 191 case UNABLETOASSESS: 192 tgt.setValue(org.hl7.fhir.dstu3.model.AllergyIntolerance.AllergyIntoleranceCriticality.UNABLETOASSESS); 193 break; 194 default: 195 tgt.setValue(org.hl7.fhir.dstu3.model.AllergyIntolerance.AllergyIntoleranceCriticality.NULL); 196 break; 197 } 198 return tgt; 199 } 200 201 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.AllergyIntolerance.AllergyIntoleranceCriticality> convertAllergyIntoleranceCriticality(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.AllergyIntolerance.AllergyIntoleranceCriticality> src) throws FHIRException { 202 if (src == null || src.isEmpty()) 203 return null; 204 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.AllergyIntolerance.AllergyIntoleranceCriticality> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.AllergyIntolerance.AllergyIntoleranceCriticalityEnumFactory()); 205 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 206 switch (src.getValue()) { 207 case LOW: 208 tgt.setValue(org.hl7.fhir.r5.model.AllergyIntolerance.AllergyIntoleranceCriticality.LOW); 209 break; 210 case HIGH: 211 tgt.setValue(org.hl7.fhir.r5.model.AllergyIntolerance.AllergyIntoleranceCriticality.HIGH); 212 break; 213 case UNABLETOASSESS: 214 tgt.setValue(org.hl7.fhir.r5.model.AllergyIntolerance.AllergyIntoleranceCriticality.UNABLETOASSESS); 215 break; 216 default: 217 tgt.setValue(org.hl7.fhir.r5.model.AllergyIntolerance.AllergyIntoleranceCriticality.NULL); 218 break; 219 } 220 return tgt; 221 } 222 223 public static org.hl7.fhir.dstu3.model.AllergyIntolerance.AllergyIntoleranceReactionComponent convertAllergyIntoleranceReactionComponent(org.hl7.fhir.r5.model.AllergyIntolerance.AllergyIntoleranceReactionComponent src) throws FHIRException { 224 if (src == null) 225 return null; 226 org.hl7.fhir.dstu3.model.AllergyIntolerance.AllergyIntoleranceReactionComponent tgt = new org.hl7.fhir.dstu3.model.AllergyIntolerance.AllergyIntoleranceReactionComponent(); 227 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 228 if (src.hasSubstance()) 229 tgt.setSubstance(CodeableConcept30_50.convertCodeableConcept(src.getSubstance())); 230 for (CodeableReference t : src.getManifestation()) 231 if (t.hasConcept()) tgt.addManifestation(CodeableConcept30_50.convertCodeableConcept(t.getConcept())); 232 if (src.hasDescription()) 233 tgt.setDescriptionElement(String30_50.convertString(src.getDescriptionElement())); 234 if (src.hasOnset()) 235 tgt.setOnsetElement(DateTime30_50.convertDateTime(src.getOnsetElement())); 236 if (src.hasSeverity()) 237 tgt.setSeverityElement(convertAllergyIntoleranceSeverity(src.getSeverityElement())); 238 if (src.hasExposureRoute()) 239 tgt.setExposureRoute(CodeableConcept30_50.convertCodeableConcept(src.getExposureRoute())); 240 for (org.hl7.fhir.r5.model.Annotation t : src.getNote()) tgt.addNote(Annotation30_50.convertAnnotation(t)); 241 return tgt; 242 } 243 244 public static org.hl7.fhir.r5.model.AllergyIntolerance.AllergyIntoleranceReactionComponent convertAllergyIntoleranceReactionComponent(org.hl7.fhir.dstu3.model.AllergyIntolerance.AllergyIntoleranceReactionComponent src) throws FHIRException { 245 if (src == null) 246 return null; 247 org.hl7.fhir.r5.model.AllergyIntolerance.AllergyIntoleranceReactionComponent tgt = new org.hl7.fhir.r5.model.AllergyIntolerance.AllergyIntoleranceReactionComponent(); 248 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 249 if (src.hasSubstance()) 250 tgt.setSubstance(CodeableConcept30_50.convertCodeableConcept(src.getSubstance())); 251 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getManifestation()) 252 tgt.addManifestation(new CodeableReference(CodeableConcept30_50.convertCodeableConcept(t))); 253 if (src.hasDescription()) 254 tgt.setDescriptionElement(String30_50.convertString(src.getDescriptionElement())); 255 if (src.hasOnset()) 256 tgt.setOnsetElement(DateTime30_50.convertDateTime(src.getOnsetElement())); 257 if (src.hasSeverity()) 258 tgt.setSeverityElement(convertAllergyIntoleranceSeverity(src.getSeverityElement())); 259 if (src.hasExposureRoute()) 260 tgt.setExposureRoute(CodeableConcept30_50.convertCodeableConcept(src.getExposureRoute())); 261 for (org.hl7.fhir.dstu3.model.Annotation t : src.getNote()) tgt.addNote(Annotation30_50.convertAnnotation(t)); 262 return tgt; 263 } 264 265 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.AllergyIntolerance.AllergyIntoleranceSeverity> convertAllergyIntoleranceSeverity(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.AllergyIntolerance.AllergyIntoleranceSeverity> src) throws FHIRException { 266 if (src == null || src.isEmpty()) 267 return null; 268 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.AllergyIntolerance.AllergyIntoleranceSeverity> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.AllergyIntolerance.AllergyIntoleranceSeverityEnumFactory()); 269 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 270 switch (src.getValue()) { 271 case MILD: 272 tgt.setValue(org.hl7.fhir.r5.model.AllergyIntolerance.AllergyIntoleranceSeverity.MILD); 273 break; 274 case MODERATE: 275 tgt.setValue(org.hl7.fhir.r5.model.AllergyIntolerance.AllergyIntoleranceSeverity.MODERATE); 276 break; 277 case SEVERE: 278 tgt.setValue(org.hl7.fhir.r5.model.AllergyIntolerance.AllergyIntoleranceSeverity.SEVERE); 279 break; 280 default: 281 tgt.setValue(org.hl7.fhir.r5.model.AllergyIntolerance.AllergyIntoleranceSeverity.NULL); 282 break; 283 } 284 return tgt; 285 } 286 287 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.AllergyIntolerance.AllergyIntoleranceSeverity> convertAllergyIntoleranceSeverity(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.AllergyIntolerance.AllergyIntoleranceSeverity> src) throws FHIRException { 288 if (src == null || src.isEmpty()) 289 return null; 290 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.AllergyIntolerance.AllergyIntoleranceSeverity> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.AllergyIntolerance.AllergyIntoleranceSeverityEnumFactory()); 291 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 292 switch (src.getValue()) { 293 case MILD: 294 tgt.setValue(org.hl7.fhir.dstu3.model.AllergyIntolerance.AllergyIntoleranceSeverity.MILD); 295 break; 296 case MODERATE: 297 tgt.setValue(org.hl7.fhir.dstu3.model.AllergyIntolerance.AllergyIntoleranceSeverity.MODERATE); 298 break; 299 case SEVERE: 300 tgt.setValue(org.hl7.fhir.dstu3.model.AllergyIntolerance.AllergyIntoleranceSeverity.SEVERE); 301 break; 302 default: 303 tgt.setValue(org.hl7.fhir.dstu3.model.AllergyIntolerance.AllergyIntoleranceSeverity.NULL); 304 break; 305 } 306 return tgt; 307 } 308 309 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.AllergyIntolerance.AllergyIntoleranceType> convertAllergyIntoleranceType(org.hl7.fhir.r5.model.CodeableConcept src) throws FHIRException { 310 if (src == null || src.isEmpty()) 311 return null; 312 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.AllergyIntolerance.AllergyIntoleranceType> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.AllergyIntolerance.AllergyIntoleranceTypeEnumFactory()); 313 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 314 if (src.hasCoding("http://hl7.org/fhir/allergy-intolerance-type", "allergy")) { 315 tgt.setValue(org.hl7.fhir.dstu3.model.AllergyIntolerance.AllergyIntoleranceType.ALLERGY); 316 } else if (src.hasCoding("http://hl7.org/fhir/allergy-intolerance-type", "intolerance")) { 317 tgt.setValue(org.hl7.fhir.dstu3.model.AllergyIntolerance.AllergyIntoleranceType.INTOLERANCE); 318 } 319 return tgt; 320 } 321 322 static public org.hl7.fhir.r5.model.CodeableConcept convertAllergyIntoleranceType(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.AllergyIntolerance.AllergyIntoleranceType> src) throws FHIRException { 323 if (src == null || src.isEmpty()) 324 return null; 325 org.hl7.fhir.r5.model.CodeableConcept tgt = new org.hl7.fhir.r5.model.CodeableConcept(); 326 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 327 switch (src.getValue()) { 328 case ALLERGY: 329 tgt.addCoding("http://hl7.org/fhir/allergy-intolerance-type", "allergy", "Allergy"); 330 break; 331 case INTOLERANCE: 332 tgt.addCoding("http://hl7.org/fhir/allergy-intolerance-type", "intolerance", "Intolerance"); 333 break; 334 default: 335 break; 336 } 337 return tgt; 338 } 339 340 static public org.hl7.fhir.r5.model.CodeableConcept convertAllergyIntoleranceVerificationStatus(org.hl7.fhir.dstu3.model.AllergyIntolerance.AllergyIntoleranceVerificationStatus src) throws FHIRException { 341 if (src == null) 342 return null; 343 switch (src) { 344 case UNCONFIRMED: 345 return new org.hl7.fhir.r5.model.CodeableConcept(new org.hl7.fhir.r5.model.Coding().setSystem("http://terminology.hl7.org/CodeSystem/allergyintolerance-verification").setCode("unconfirmed")); 346 case CONFIRMED: 347 return new org.hl7.fhir.r5.model.CodeableConcept(new org.hl7.fhir.r5.model.Coding().setSystem("http://terminology.hl7.org/CodeSystem/allergyintolerance-verification").setCode("confirmed")); 348 case REFUTED: 349 return new org.hl7.fhir.r5.model.CodeableConcept(new org.hl7.fhir.r5.model.Coding().setSystem("http://terminology.hl7.org/CodeSystem/allergyintolerance-verification").setCode("refuted")); 350 case ENTEREDINERROR: 351 return new org.hl7.fhir.r5.model.CodeableConcept(new org.hl7.fhir.r5.model.Coding().setSystem("http://terminology.hl7.org/CodeSystem/allergyintolerance-verification").setCode("entered-in-error")); 352 default: 353 return null; 354 } 355 } 356 357 static public org.hl7.fhir.dstu3.model.AllergyIntolerance.AllergyIntoleranceVerificationStatus convertAllergyIntoleranceVerificationStatus(org.hl7.fhir.r5.model.CodeableConcept src) throws FHIRException { 358 if (src == null) 359 return null; 360 if (src.hasCoding("http://terminology.hl7.org/CodeSystem/allergyintolerance-verification", "unconfirmed")) 361 return org.hl7.fhir.dstu3.model.AllergyIntolerance.AllergyIntoleranceVerificationStatus.UNCONFIRMED; 362 if (src.hasCoding("http://terminology.hl7.org/CodeSystem/allergyintolerance-verification", "confirmed")) 363 return org.hl7.fhir.dstu3.model.AllergyIntolerance.AllergyIntoleranceVerificationStatus.CONFIRMED; 364 if (src.hasCoding("http://terminology.hl7.org/CodeSystem/allergyintolerance-verification", "refuted")) 365 return org.hl7.fhir.dstu3.model.AllergyIntolerance.AllergyIntoleranceVerificationStatus.REFUTED; 366 if (src.hasCoding("http://terminology.hl7.org/CodeSystem/allergyintolerance-verification", "entered-in-error")) 367 return org.hl7.fhir.dstu3.model.AllergyIntolerance.AllergyIntoleranceVerificationStatus.ENTEREDINERROR; 368 return org.hl7.fhir.dstu3.model.AllergyIntolerance.AllergyIntoleranceVerificationStatus.NULL; 369 } 370}