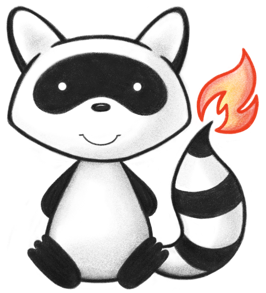
001package org.hl7.fhir.convertors.conv30_50.datatypes30_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext30_50; 004import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.CodeableConcept30_50; 005import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.Identifier30_50; 006import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.String30_50; 007import org.hl7.fhir.exceptions.FHIRException; 008 009public class Reference30_50 { 010 public static org.hl7.fhir.r5.model.Reference convertReference(org.hl7.fhir.dstu3.model.Reference src) throws FHIRException { 011 if (src == null) return null; 012 org.hl7.fhir.r5.model.Reference tgt = new org.hl7.fhir.r5.model.Reference(); 013 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 014 if (src.hasReference()) tgt.setReference(src.getReference()); 015 if (src.hasIdentifier()) tgt.setIdentifier(Identifier30_50.convertIdentifier(src.getIdentifier())); 016 if (src.hasDisplay()) tgt.setDisplayElement(String30_50.convertString(src.getDisplayElement())); 017 return tgt; 018 } 019 020 public static org.hl7.fhir.dstu3.model.Reference convertReference(org.hl7.fhir.r5.model.Reference src) throws FHIRException { 021 if (src == null) return null; 022 org.hl7.fhir.dstu3.model.Reference tgt = new org.hl7.fhir.dstu3.model.Reference(); 023 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 024 if (src.hasReference()) tgt.setReference(src.getReference()); 025 if (src.hasIdentifier()) tgt.setIdentifier(Identifier30_50.convertIdentifier(src.getIdentifier())); 026 if (src.hasDisplay()) tgt.setDisplayElement(String30_50.convertString(src.getDisplayElement())); 027 return tgt; 028 } 029 030 static public org.hl7.fhir.r5.model.CodeableReference convertReferenceToCodableReference(org.hl7.fhir.dstu3.model.Reference src) { 031 org.hl7.fhir.r5.model.CodeableReference tgt = new org.hl7.fhir.r5.model.CodeableReference(); 032 tgt.setReference(convertReference(src)); 033 return tgt; 034 } 035 036 static public org.hl7.fhir.dstu3.model.Reference convertCodeableReferenceToReference(org.hl7.fhir.r5.model.CodeableReference src) { 037 org.hl7.fhir.dstu3.model.Reference tgt = new org.hl7.fhir.dstu3.model.Reference(); 038 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 039 tgt.setReference(src.getReference().getReference()); 040 return tgt; 041 } 042 043 static public org.hl7.fhir.r5.model.CodeableReference convertCodeableConceptToCodableReference(org.hl7.fhir.dstu3.model.CodeableConcept src) { 044 org.hl7.fhir.r5.model.CodeableReference tgt = new org.hl7.fhir.r5.model.CodeableReference(); 045 tgt.setConcept(CodeableConcept30_50.convertCodeableConcept(src)); 046 return tgt; 047 } 048 049 static public org.hl7.fhir.r5.model.CanonicalType convertReferenceToCanonical(org.hl7.fhir.dstu3.model.Reference src) throws FHIRException { 050 org.hl7.fhir.r5.model.CanonicalType dst = new org.hl7.fhir.r5.model.CanonicalType(src.getReference()); 051 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, dst); 052 return dst; 053 } 054 055 static public org.hl7.fhir.dstu3.model.Reference convertCanonicalToReference(org.hl7.fhir.r5.model.CanonicalType src) throws FHIRException { 056 org.hl7.fhir.dstu3.model.Reference dst = new org.hl7.fhir.dstu3.model.Reference(src.getValue()); 057 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, dst); 058 return dst; 059 } 060}