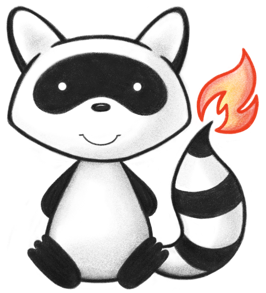
001package org.hl7.fhir.convertors.conv30_40.resources30_40; 002 003import org.hl7.fhir.convertors.context.ConversionContext30_40; 004import org.hl7.fhir.convertors.conv30_40.datatypes30_40.Reference30_40; 005import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.CodeableConcept30_40; 006import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.Coding30_40; 007import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.ContactPoint30_40; 008import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.Identifier30_40; 009import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.Period30_40; 010import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.String30_40; 011import org.hl7.fhir.exceptions.FHIRException; 012 013public class Endpoint30_40 { 014 015 public static org.hl7.fhir.r4.model.Endpoint convertEndpoint(org.hl7.fhir.dstu3.model.Endpoint src) throws FHIRException { 016 if (src == null) 017 return null; 018 org.hl7.fhir.r4.model.Endpoint tgt = new org.hl7.fhir.r4.model.Endpoint(); 019 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyDomainResource(src, tgt); 020 for (org.hl7.fhir.dstu3.model.Identifier t : src.getIdentifier()) 021 tgt.addIdentifier(Identifier30_40.convertIdentifier(t)); 022 if (src.hasStatus()) 023 tgt.setStatusElement(convertEndpointStatus(src.getStatusElement())); 024 if (src.hasConnectionType()) 025 tgt.setConnectionType(Coding30_40.convertCoding(src.getConnectionType())); 026 if (src.hasName()) 027 tgt.setNameElement(String30_40.convertString(src.getNameElement())); 028 if (src.hasManagingOrganization()) 029 tgt.setManagingOrganization(Reference30_40.convertReference(src.getManagingOrganization())); 030 for (org.hl7.fhir.dstu3.model.ContactPoint t : src.getContact()) 031 tgt.addContact(ContactPoint30_40.convertContactPoint(t)); 032 if (src.hasPeriod()) 033 tgt.setPeriod(Period30_40.convertPeriod(src.getPeriod())); 034 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getPayloadType()) 035 tgt.addPayloadType(CodeableConcept30_40.convertCodeableConcept(t)); 036 for (org.hl7.fhir.dstu3.model.CodeType t : src.getPayloadMimeType()) tgt.addPayloadMimeType(t.getValue()); 037 if (src.hasAddress()) 038 tgt.setAddress(src.getAddress()); 039 for (org.hl7.fhir.dstu3.model.StringType t : src.getHeader()) tgt.addHeader(t.getValue()); 040 return tgt; 041 } 042 043 public static org.hl7.fhir.dstu3.model.Endpoint convertEndpoint(org.hl7.fhir.r4.model.Endpoint src) throws FHIRException { 044 if (src == null) 045 return null; 046 org.hl7.fhir.dstu3.model.Endpoint tgt = new org.hl7.fhir.dstu3.model.Endpoint(); 047 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyDomainResource(src, tgt); 048 for (org.hl7.fhir.r4.model.Identifier t : src.getIdentifier()) 049 tgt.addIdentifier(Identifier30_40.convertIdentifier(t)); 050 if (src.hasStatus()) 051 tgt.setStatusElement(convertEndpointStatus(src.getStatusElement())); 052 if (src.hasConnectionType()) 053 tgt.setConnectionType(Coding30_40.convertCoding(src.getConnectionType())); 054 if (src.hasName()) 055 tgt.setNameElement(String30_40.convertString(src.getNameElement())); 056 if (src.hasManagingOrganization()) 057 tgt.setManagingOrganization(Reference30_40.convertReference(src.getManagingOrganization())); 058 for (org.hl7.fhir.r4.model.ContactPoint t : src.getContact()) 059 tgt.addContact(ContactPoint30_40.convertContactPoint(t)); 060 if (src.hasPeriod()) 061 tgt.setPeriod(Period30_40.convertPeriod(src.getPeriod())); 062 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getPayloadType()) 063 tgt.addPayloadType(CodeableConcept30_40.convertCodeableConcept(t)); 064 for (org.hl7.fhir.r4.model.CodeType t : src.getPayloadMimeType()) tgt.addPayloadMimeType(t.getValue()); 065 if (src.hasAddress()) 066 tgt.setAddress(src.getAddress()); 067 for (org.hl7.fhir.r4.model.StringType t : src.getHeader()) tgt.addHeader(t.getValue()); 068 return tgt; 069 } 070 071 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Endpoint.EndpointStatus> convertEndpointStatus(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Endpoint.EndpointStatus> src) throws FHIRException { 072 if (src == null || src.isEmpty()) 073 return null; 074 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Endpoint.EndpointStatus> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.Endpoint.EndpointStatusEnumFactory()); 075 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 076 switch (src.getValue()) { 077 case ACTIVE: 078 tgt.setValue(org.hl7.fhir.r4.model.Endpoint.EndpointStatus.ACTIVE); 079 break; 080 case SUSPENDED: 081 tgt.setValue(org.hl7.fhir.r4.model.Endpoint.EndpointStatus.SUSPENDED); 082 break; 083 case ERROR: 084 tgt.setValue(org.hl7.fhir.r4.model.Endpoint.EndpointStatus.ERROR); 085 break; 086 case OFF: 087 tgt.setValue(org.hl7.fhir.r4.model.Endpoint.EndpointStatus.OFF); 088 break; 089 case ENTEREDINERROR: 090 tgt.setValue(org.hl7.fhir.r4.model.Endpoint.EndpointStatus.ENTEREDINERROR); 091 break; 092 case TEST: 093 tgt.setValue(org.hl7.fhir.r4.model.Endpoint.EndpointStatus.TEST); 094 break; 095 default: 096 tgt.setValue(org.hl7.fhir.r4.model.Endpoint.EndpointStatus.NULL); 097 break; 098 } 099 return tgt; 100 } 101 102 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Endpoint.EndpointStatus> convertEndpointStatus(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Endpoint.EndpointStatus> src) throws FHIRException { 103 if (src == null || src.isEmpty()) 104 return null; 105 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Endpoint.EndpointStatus> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.Endpoint.EndpointStatusEnumFactory()); 106 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 107 switch (src.getValue()) { 108 case ACTIVE: 109 tgt.setValue(org.hl7.fhir.dstu3.model.Endpoint.EndpointStatus.ACTIVE); 110 break; 111 case SUSPENDED: 112 tgt.setValue(org.hl7.fhir.dstu3.model.Endpoint.EndpointStatus.SUSPENDED); 113 break; 114 case ERROR: 115 tgt.setValue(org.hl7.fhir.dstu3.model.Endpoint.EndpointStatus.ERROR); 116 break; 117 case OFF: 118 tgt.setValue(org.hl7.fhir.dstu3.model.Endpoint.EndpointStatus.OFF); 119 break; 120 case ENTEREDINERROR: 121 tgt.setValue(org.hl7.fhir.dstu3.model.Endpoint.EndpointStatus.ENTEREDINERROR); 122 break; 123 case TEST: 124 tgt.setValue(org.hl7.fhir.dstu3.model.Endpoint.EndpointStatus.TEST); 125 break; 126 default: 127 tgt.setValue(org.hl7.fhir.dstu3.model.Endpoint.EndpointStatus.NULL); 128 break; 129 } 130 return tgt; 131 } 132}