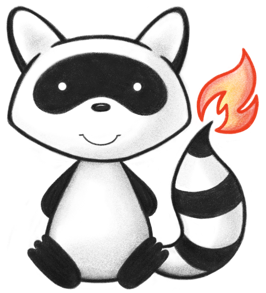
001package org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40; 002 003import java.util.stream.Collectors; 004 005import org.hl7.fhir.convertors.context.ConversionContext30_40; 006import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.Decimal30_40; 007import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.UnsignedInt30_40; 008import org.hl7.fhir.exceptions.FHIRException; 009 010public class Timing30_40 { 011 public static org.hl7.fhir.r4.model.Timing convertTiming(org.hl7.fhir.dstu3.model.Timing src) throws FHIRException { 012 if (src == null) return null; 013 org.hl7.fhir.r4.model.Timing tgt = new org.hl7.fhir.r4.model.Timing(); 014 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 015 for (org.hl7.fhir.dstu3.model.DateTimeType t : src.getEvent()) 016 tgt.addEventElement().setValueAsString(t.getValueAsString()); 017 if (src.hasRepeat()) tgt.setRepeat(convertTimingRepeatComponent(src.getRepeat())); 018 if (src.hasCode()) tgt.setCode(CodeableConcept30_40.convertCodeableConcept(src.getCode())); 019 return tgt; 020 } 021 022 public static org.hl7.fhir.dstu3.model.Timing convertTiming(org.hl7.fhir.r4.model.Timing src) throws FHIRException { 023 if (src == null) return null; 024 org.hl7.fhir.dstu3.model.Timing tgt = new org.hl7.fhir.dstu3.model.Timing(); 025 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 026 for (org.hl7.fhir.r4.model.DateTimeType t : src.getEvent()) 027 tgt.addEventElement().setValueAsString(t.getValueAsString()); 028 if (src.hasRepeat()) tgt.setRepeat(convertTimingRepeatComponent(src.getRepeat())); 029 if (src.hasCode()) tgt.setCode(CodeableConcept30_40.convertCodeableConcept(src.getCode())); 030 return tgt; 031 } 032 033 public static org.hl7.fhir.r4.model.Timing.TimingRepeatComponent convertTimingRepeatComponent(org.hl7.fhir.dstu3.model.Timing.TimingRepeatComponent src) throws FHIRException { 034 if (src == null) return null; 035 org.hl7.fhir.r4.model.Timing.TimingRepeatComponent tgt = new org.hl7.fhir.r4.model.Timing.TimingRepeatComponent(); 036 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 037 if (src.hasBounds()) 038 tgt.setBounds(ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().convertType(src.getBounds())); 039 if (src.hasCount()) tgt.setCount(src.getCount()); 040 if (src.hasCountMax()) tgt.setCountMax(src.getCountMax()); 041 if (src.hasDuration()) tgt.setDurationElement(Decimal30_40.convertDecimal(src.getDurationElement())); 042 if (src.hasDurationMax()) tgt.setDurationMaxElement(Decimal30_40.convertDecimal(src.getDurationMaxElement())); 043 if (src.hasDurationUnit()) tgt.setDurationUnitElement(convertUnitsOfTime(src.getDurationUnitElement())); 044 if (src.hasFrequency()) tgt.setFrequency(src.getFrequency()); 045 if (src.hasFrequencyMax()) tgt.setFrequencyMax(src.getFrequencyMax()); 046 if (src.hasPeriod()) tgt.setPeriodElement(Decimal30_40.convertDecimal(src.getPeriodElement())); 047 if (src.hasPeriodMax()) tgt.setPeriodMaxElement(Decimal30_40.convertDecimal(src.getPeriodMaxElement())); 048 if (src.hasPeriodUnit()) tgt.setPeriodUnitElement(convertUnitsOfTime(src.getPeriodUnitElement())); 049 tgt.setDayOfWeek(src.getDayOfWeek().stream().map(Timing30_40::convertDayOfWeek).collect(Collectors.toList())); 050 for (org.hl7.fhir.dstu3.model.TimeType t : src.getTimeOfDay()) tgt.addTimeOfDay(t.getValue()); 051 if (src.hasWhen()) 052 tgt.setWhen(src.getWhen().stream().map(Timing30_40::convertEventTiming).collect(Collectors.toList())); 053 if (src.hasOffset()) tgt.setOffsetElement(UnsignedInt30_40.convertUnsignedInt(src.getOffsetElement())); 054 return tgt; 055 } 056 057 public static org.hl7.fhir.dstu3.model.Timing.TimingRepeatComponent convertTimingRepeatComponent(org.hl7.fhir.r4.model.Timing.TimingRepeatComponent src) throws FHIRException { 058 if (src == null) return null; 059 org.hl7.fhir.dstu3.model.Timing.TimingRepeatComponent tgt = new org.hl7.fhir.dstu3.model.Timing.TimingRepeatComponent(); 060 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 061 if (src.hasBounds()) 062 tgt.setBounds(ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().convertType(src.getBounds())); 063 if (src.hasCount()) tgt.setCount(src.getCount()); 064 if (src.hasCountMax()) tgt.setCountMax(src.getCountMax()); 065 if (src.hasDuration()) tgt.setDurationElement(Decimal30_40.convertDecimal(src.getDurationElement())); 066 if (src.hasDurationMax()) tgt.setDurationMaxElement(Decimal30_40.convertDecimal(src.getDurationMaxElement())); 067 if (src.hasDurationUnit()) tgt.setDurationUnitElement(convertUnitsOfTime(src.getDurationUnitElement())); 068 if (src.hasFrequency()) tgt.setFrequency(src.getFrequency()); 069 if (src.hasFrequencyMax()) tgt.setFrequencyMax(src.getFrequencyMax()); 070 if (src.hasPeriod()) tgt.setPeriodElement(Decimal30_40.convertDecimal(src.getPeriodElement())); 071 if (src.hasPeriodMax()) tgt.setPeriodMaxElement(Decimal30_40.convertDecimal(src.getPeriodMaxElement())); 072 if (src.hasPeriodUnit()) tgt.setPeriodUnitElement(convertUnitsOfTime(src.getPeriodUnitElement())); 073 tgt.setDayOfWeek(src.getDayOfWeek().stream().map(Timing30_40::convertDayOfWeek).collect(Collectors.toList())); 074 for (org.hl7.fhir.r4.model.TimeType t : src.getTimeOfDay()) tgt.addTimeOfDay(t.getValue()); 075 if (src.hasWhen()) 076 tgt.setWhen(src.getWhen().stream().map(Timing30_40::convertEventTiming).collect(Collectors.toList())); 077 if (src.hasOffset()) tgt.setOffsetElement(UnsignedInt30_40.convertUnsignedInt(src.getOffsetElement())); 078 return tgt; 079 } 080 081 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Timing.EventTiming> convertEventTiming(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Timing.EventTiming> src) throws FHIRException { 082 if (src == null || src.isEmpty()) return null; 083 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Timing.EventTiming> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.Timing.EventTimingEnumFactory()); 084 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 085 if (src.getValue() == null) { 086 tgt.setValue(org.hl7.fhir.r4.model.Timing.EventTiming.NULL); 087 } else { 088 switch (src.getValue()) { 089 case MORN: 090 tgt.setValue(org.hl7.fhir.r4.model.Timing.EventTiming.MORN); 091 break; 092 case AFT: 093 tgt.setValue(org.hl7.fhir.r4.model.Timing.EventTiming.AFT); 094 break; 095 case EVE: 096 tgt.setValue(org.hl7.fhir.r4.model.Timing.EventTiming.EVE); 097 break; 098 case NIGHT: 099 tgt.setValue(org.hl7.fhir.r4.model.Timing.EventTiming.NIGHT); 100 break; 101 case PHS: 102 tgt.setValue(org.hl7.fhir.r4.model.Timing.EventTiming.PHS); 103 break; 104 case HS: 105 tgt.setValue(org.hl7.fhir.r4.model.Timing.EventTiming.HS); 106 break; 107 case WAKE: 108 tgt.setValue(org.hl7.fhir.r4.model.Timing.EventTiming.WAKE); 109 break; 110 case C: 111 tgt.setValue(org.hl7.fhir.r4.model.Timing.EventTiming.C); 112 break; 113 case CM: 114 tgt.setValue(org.hl7.fhir.r4.model.Timing.EventTiming.CM); 115 break; 116 case CD: 117 tgt.setValue(org.hl7.fhir.r4.model.Timing.EventTiming.CD); 118 break; 119 case CV: 120 tgt.setValue(org.hl7.fhir.r4.model.Timing.EventTiming.CV); 121 break; 122 case AC: 123 tgt.setValue(org.hl7.fhir.r4.model.Timing.EventTiming.AC); 124 break; 125 case ACM: 126 tgt.setValue(org.hl7.fhir.r4.model.Timing.EventTiming.ACM); 127 break; 128 case ACD: 129 tgt.setValue(org.hl7.fhir.r4.model.Timing.EventTiming.ACD); 130 break; 131 case ACV: 132 tgt.setValue(org.hl7.fhir.r4.model.Timing.EventTiming.ACV); 133 break; 134 case PC: 135 tgt.setValue(org.hl7.fhir.r4.model.Timing.EventTiming.PC); 136 break; 137 case PCM: 138 tgt.setValue(org.hl7.fhir.r4.model.Timing.EventTiming.PCM); 139 break; 140 case PCD: 141 tgt.setValue(org.hl7.fhir.r4.model.Timing.EventTiming.PCD); 142 break; 143 case PCV: 144 tgt.setValue(org.hl7.fhir.r4.model.Timing.EventTiming.PCV); 145 break; 146 default: 147 tgt.setValue(org.hl7.fhir.r4.model.Timing.EventTiming.NULL); 148 break; 149 } 150 } 151 return tgt; 152 } 153 154 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Timing.EventTiming> convertEventTiming(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Timing.EventTiming> src) throws FHIRException { 155 if (src == null || src.isEmpty()) return null; 156 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Timing.EventTiming> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.Timing.EventTimingEnumFactory()); 157 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 158 if (src.getValue() == null) { 159 tgt.setValue(org.hl7.fhir.dstu3.model.Timing.EventTiming.NULL); 160 } else { 161 switch (src.getValue()) { 162 case MORN: 163 tgt.setValue(org.hl7.fhir.dstu3.model.Timing.EventTiming.MORN); 164 break; 165 case AFT: 166 tgt.setValue(org.hl7.fhir.dstu3.model.Timing.EventTiming.AFT); 167 break; 168 case EVE: 169 tgt.setValue(org.hl7.fhir.dstu3.model.Timing.EventTiming.EVE); 170 break; 171 case NIGHT: 172 tgt.setValue(org.hl7.fhir.dstu3.model.Timing.EventTiming.NIGHT); 173 break; 174 case PHS: 175 tgt.setValue(org.hl7.fhir.dstu3.model.Timing.EventTiming.PHS); 176 break; 177 case HS: 178 tgt.setValue(org.hl7.fhir.dstu3.model.Timing.EventTiming.HS); 179 break; 180 case WAKE: 181 tgt.setValue(org.hl7.fhir.dstu3.model.Timing.EventTiming.WAKE); 182 break; 183 case C: 184 tgt.setValue(org.hl7.fhir.dstu3.model.Timing.EventTiming.C); 185 break; 186 case CM: 187 tgt.setValue(org.hl7.fhir.dstu3.model.Timing.EventTiming.CM); 188 break; 189 case CD: 190 tgt.setValue(org.hl7.fhir.dstu3.model.Timing.EventTiming.CD); 191 break; 192 case CV: 193 tgt.setValue(org.hl7.fhir.dstu3.model.Timing.EventTiming.CV); 194 break; 195 case AC: 196 tgt.setValue(org.hl7.fhir.dstu3.model.Timing.EventTiming.AC); 197 break; 198 case ACM: 199 tgt.setValue(org.hl7.fhir.dstu3.model.Timing.EventTiming.ACM); 200 break; 201 case ACD: 202 tgt.setValue(org.hl7.fhir.dstu3.model.Timing.EventTiming.ACD); 203 break; 204 case ACV: 205 tgt.setValue(org.hl7.fhir.dstu3.model.Timing.EventTiming.ACV); 206 break; 207 case PC: 208 tgt.setValue(org.hl7.fhir.dstu3.model.Timing.EventTiming.PC); 209 break; 210 case PCM: 211 tgt.setValue(org.hl7.fhir.dstu3.model.Timing.EventTiming.PCM); 212 break; 213 case PCD: 214 tgt.setValue(org.hl7.fhir.dstu3.model.Timing.EventTiming.PCD); 215 break; 216 case PCV: 217 tgt.setValue(org.hl7.fhir.dstu3.model.Timing.EventTiming.PCV); 218 break; 219 default: 220 tgt.setValue(org.hl7.fhir.dstu3.model.Timing.EventTiming.NULL); 221 break; 222 } 223 } 224 return tgt; 225 } 226 227 public static org.hl7.fhir.r4.model.UsageContext convertUsageContext(org.hl7.fhir.dstu3.model.UsageContext src) throws FHIRException { 228 if (src == null) return null; 229 org.hl7.fhir.r4.model.UsageContext tgt = new org.hl7.fhir.r4.model.UsageContext(); 230 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 231 if (src.hasCode()) tgt.setCode(Coding30_40.convertCoding(src.getCode())); 232 if (src.hasValue()) 233 tgt.setValue(ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().convertType(src.getValue())); 234 return tgt; 235 } 236 237 public static org.hl7.fhir.dstu3.model.UsageContext convertUsageContext(org.hl7.fhir.r4.model.UsageContext src) throws FHIRException { 238 if (src == null) return null; 239 org.hl7.fhir.dstu3.model.UsageContext tgt = new org.hl7.fhir.dstu3.model.UsageContext(); 240 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 241 if (src.hasCode()) tgt.setCode(Coding30_40.convertCoding(src.getCode())); 242 if (src.hasValue()) 243 tgt.setValue(ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().convertType(src.getValue())); 244 return tgt; 245 } 246 247 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Timing.DayOfWeek> convertDayOfWeek(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Timing.DayOfWeek> src) throws FHIRException { 248 if (src == null || src.isEmpty()) return null; 249 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Timing.DayOfWeek> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.Timing.DayOfWeekEnumFactory()); 250 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 251 if (src.getValue() == null) { 252 tgt.setValue(org.hl7.fhir.dstu3.model.Timing.DayOfWeek.NULL); 253 } else { 254 switch (src.getValue()) { 255 case MON: 256 tgt.setValue(org.hl7.fhir.dstu3.model.Timing.DayOfWeek.MON); 257 break; 258 case TUE: 259 tgt.setValue(org.hl7.fhir.dstu3.model.Timing.DayOfWeek.TUE); 260 break; 261 case WED: 262 tgt.setValue(org.hl7.fhir.dstu3.model.Timing.DayOfWeek.WED); 263 break; 264 case THU: 265 tgt.setValue(org.hl7.fhir.dstu3.model.Timing.DayOfWeek.THU); 266 break; 267 case FRI: 268 tgt.setValue(org.hl7.fhir.dstu3.model.Timing.DayOfWeek.FRI); 269 break; 270 case SAT: 271 tgt.setValue(org.hl7.fhir.dstu3.model.Timing.DayOfWeek.SAT); 272 break; 273 case SUN: 274 tgt.setValue(org.hl7.fhir.dstu3.model.Timing.DayOfWeek.SUN); 275 break; 276 default: 277 tgt.setValue(org.hl7.fhir.dstu3.model.Timing.DayOfWeek.NULL); 278 break; 279 } 280 } 281 return tgt; 282 } 283 284 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Timing.UnitsOfTime> convertUnitsOfTime(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Timing.UnitsOfTime> src) throws FHIRException { 285 if (src == null || src.isEmpty()) return null; 286 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Timing.UnitsOfTime> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.Timing.UnitsOfTimeEnumFactory()); 287 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 288 if (src.getValue() == null) { 289 tgt.setValue(org.hl7.fhir.r4.model.Timing.UnitsOfTime.NULL); 290 } else { 291 switch (src.getValue()) { 292 case S: 293 tgt.setValue(org.hl7.fhir.r4.model.Timing.UnitsOfTime.S); 294 break; 295 case MIN: 296 tgt.setValue(org.hl7.fhir.r4.model.Timing.UnitsOfTime.MIN); 297 break; 298 case H: 299 tgt.setValue(org.hl7.fhir.r4.model.Timing.UnitsOfTime.H); 300 break; 301 case D: 302 tgt.setValue(org.hl7.fhir.r4.model.Timing.UnitsOfTime.D); 303 break; 304 case WK: 305 tgt.setValue(org.hl7.fhir.r4.model.Timing.UnitsOfTime.WK); 306 break; 307 case MO: 308 tgt.setValue(org.hl7.fhir.r4.model.Timing.UnitsOfTime.MO); 309 break; 310 case A: 311 tgt.setValue(org.hl7.fhir.r4.model.Timing.UnitsOfTime.A); 312 break; 313 default: 314 tgt.setValue(org.hl7.fhir.r4.model.Timing.UnitsOfTime.NULL); 315 break; 316 } 317 } 318 return tgt; 319 } 320 321 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Timing.UnitsOfTime> convertUnitsOfTime(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Timing.UnitsOfTime> src) throws FHIRException { 322 if (src == null || src.isEmpty()) return null; 323 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Timing.UnitsOfTime> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.Timing.UnitsOfTimeEnumFactory()); 324 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 325 if (src.getValue() == null) { 326 tgt.setValue(org.hl7.fhir.dstu3.model.Timing.UnitsOfTime.NULL); 327 } else { 328 switch (src.getValue()) { 329 case S: 330 tgt.setValue(org.hl7.fhir.dstu3.model.Timing.UnitsOfTime.S); 331 break; 332 case MIN: 333 tgt.setValue(org.hl7.fhir.dstu3.model.Timing.UnitsOfTime.MIN); 334 break; 335 case H: 336 tgt.setValue(org.hl7.fhir.dstu3.model.Timing.UnitsOfTime.H); 337 break; 338 case D: 339 tgt.setValue(org.hl7.fhir.dstu3.model.Timing.UnitsOfTime.D); 340 break; 341 case WK: 342 tgt.setValue(org.hl7.fhir.dstu3.model.Timing.UnitsOfTime.WK); 343 break; 344 case MO: 345 tgt.setValue(org.hl7.fhir.dstu3.model.Timing.UnitsOfTime.MO); 346 break; 347 case A: 348 tgt.setValue(org.hl7.fhir.dstu3.model.Timing.UnitsOfTime.A); 349 break; 350 default: 351 tgt.setValue(org.hl7.fhir.dstu3.model.Timing.UnitsOfTime.NULL); 352 break; 353 } 354 } 355 return tgt; 356 } 357 358 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Timing.DayOfWeek> convertDayOfWeek(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Timing.DayOfWeek> src) throws FHIRException { 359 if (src == null || src.isEmpty()) return null; 360 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Timing.DayOfWeek> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.Timing.DayOfWeekEnumFactory()); 361 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 362 if (src.getValue() == null) { 363 tgt.setValue(org.hl7.fhir.r4.model.Timing.DayOfWeek.NULL); 364 } else { 365 switch (src.getValue()) { 366 case MON: 367 tgt.setValue(org.hl7.fhir.r4.model.Timing.DayOfWeek.MON); 368 break; 369 case TUE: 370 tgt.setValue(org.hl7.fhir.r4.model.Timing.DayOfWeek.TUE); 371 break; 372 case WED: 373 tgt.setValue(org.hl7.fhir.r4.model.Timing.DayOfWeek.WED); 374 break; 375 case THU: 376 tgt.setValue(org.hl7.fhir.r4.model.Timing.DayOfWeek.THU); 377 break; 378 case FRI: 379 tgt.setValue(org.hl7.fhir.r4.model.Timing.DayOfWeek.FRI); 380 break; 381 case SAT: 382 tgt.setValue(org.hl7.fhir.r4.model.Timing.DayOfWeek.SAT); 383 break; 384 case SUN: 385 tgt.setValue(org.hl7.fhir.r4.model.Timing.DayOfWeek.SUN); 386 break; 387 default: 388 tgt.setValue(org.hl7.fhir.r4.model.Timing.DayOfWeek.NULL); 389 break; 390 } 391 } 392 return tgt; 393 } 394}