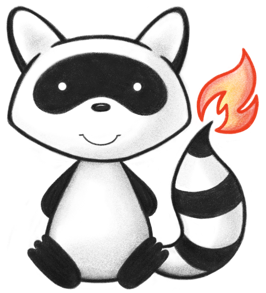
001package org.hl7.fhir.convertors.conv14_50.datatypes14_50.complextypes14_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext14_50; 004import org.hl7.fhir.convertors.conv14_50.datatypes14_50.primitivetypes14_50.String14_50; 005import org.hl7.fhir.dstu2016may.model.CodeableConcept; 006import org.hl7.fhir.exceptions.FHIRException; 007 008public class CodeableConcept14_50 { 009 public static org.hl7.fhir.r5.model.CodeableConcept convertCodeableConcept(org.hl7.fhir.dstu2016may.model.CodeableConcept src) throws FHIRException { 010 if (src == null || src.isEmpty()) return null; 011 org.hl7.fhir.r5.model.CodeableConcept tgt = new org.hl7.fhir.r5.model.CodeableConcept(); 012 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyElement(src, tgt); 013 for (org.hl7.fhir.dstu2016may.model.Coding t : src.getCoding()) tgt.addCoding(Coding14_50.convertCoding(t)); 014 if (src.hasText()) tgt.setTextElement(String14_50.convertString(src.getTextElement())); 015 return tgt; 016 } 017 018 public static org.hl7.fhir.dstu2016may.model.CodeableConcept convertCodeableConcept(org.hl7.fhir.r5.model.CodeableConcept src) throws FHIRException { 019 if (src == null || src.isEmpty()) return null; 020 org.hl7.fhir.dstu2016may.model.CodeableConcept tgt = new org.hl7.fhir.dstu2016may.model.CodeableConcept(); 021 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyElement(src, tgt); 022 for (org.hl7.fhir.r5.model.Coding t : src.getCoding()) tgt.addCoding(Coding14_50.convertCoding(t)); 023 if (src.hasText()) tgt.setTextElement(String14_50.convertString(src.getTextElement())); 024 return tgt; 025 } 026 027 static public boolean isJurisdiction(CodeableConcept t) { 028 return t.hasCoding() && ("http://unstats.un.org/unsd/methods/m49/m49.htm".equals(t.getCoding().get(0).getSystem()) || "urn:iso:std:iso:3166".equals(t.getCoding().get(0).getSystem()) || "https://www.usps.com/".equals(t.getCoding().get(0).getSystem())); 029 } 030 031 public static org.hl7.fhir.r5.model.UsageContext convertCodeableConceptToUsageContext(CodeableConcept t) throws FHIRException { 032 org.hl7.fhir.r5.model.UsageContext result = new org.hl7.fhir.r5.model.UsageContext(); 033 result.setValue(convertCodeableConcept(t)); 034 return result; 035 } 036}