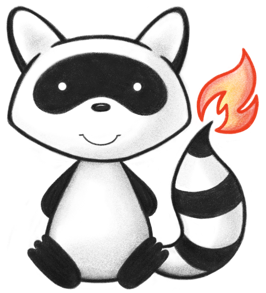
001package org.hl7.fhir.convertors.conv14_40.resources14_40; 002 003import org.hl7.fhir.convertors.VersionConvertorConstants; 004import org.hl7.fhir.convertors.context.ConversionContext14_40; 005import org.hl7.fhir.convertors.conv14_40.VersionConvertor_14_40; 006import org.hl7.fhir.convertors.conv14_40.datatypes14_40.Reference14_40; 007import org.hl7.fhir.convertors.conv14_40.datatypes14_40.complextypes14_40.CodeableConcept14_40; 008import org.hl7.fhir.convertors.conv14_40.datatypes14_40.complextypes14_40.Coding14_40; 009import org.hl7.fhir.convertors.conv14_40.datatypes14_40.complextypes14_40.ContactPoint14_40; 010import org.hl7.fhir.convertors.conv14_40.datatypes14_40.primitivetypes14_40.Boolean14_40; 011import org.hl7.fhir.convertors.conv14_40.datatypes14_40.primitivetypes14_40.Code14_40; 012import org.hl7.fhir.convertors.conv14_40.datatypes14_40.primitivetypes14_40.DateTime14_40; 013import org.hl7.fhir.convertors.conv14_40.datatypes14_40.primitivetypes14_40.String14_40; 014import org.hl7.fhir.convertors.conv14_40.datatypes14_40.primitivetypes14_40.UnsignedInt14_40; 015import org.hl7.fhir.convertors.conv14_40.datatypes14_40.primitivetypes14_40.Uri14_40; 016import org.hl7.fhir.exceptions.FHIRException; 017import org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementRestComponent; 018import org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementRestResourceComponent; 019 020public class Conformance14_40 { 021 022 public static final String ACCEPT_UNKNOWN_EXTENSION_URL = "http://hl7.org/fhir/3.0/StructureDefinition/extension-CapabilityStatement.acceptUnknown"; 023 024 private static final String[] IGNORED_EXTENSION_URLS = new String[]{ 025 ACCEPT_UNKNOWN_EXTENSION_URL 026 }; 027 028 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.CapabilityStatement.ConditionalDeleteStatus> convertConditionalDeleteStatus(org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Conformance.ConditionalDeleteStatus> src) throws FHIRException { 029 if (src == null || src.isEmpty()) 030 return null; 031 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.CapabilityStatement.ConditionalDeleteStatus> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.CapabilityStatement.ConditionalDeleteStatusEnumFactory()); 032 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyElement(src, tgt); 033 switch (src.getValue()) { 034 case NOTSUPPORTED: 035 tgt.setValue(org.hl7.fhir.r4.model.CapabilityStatement.ConditionalDeleteStatus.NOTSUPPORTED); 036 break; 037 case SINGLE: 038 tgt.setValue(org.hl7.fhir.r4.model.CapabilityStatement.ConditionalDeleteStatus.SINGLE); 039 break; 040 case MULTIPLE: 041 tgt.setValue(org.hl7.fhir.r4.model.CapabilityStatement.ConditionalDeleteStatus.MULTIPLE); 042 break; 043 default: 044 tgt.setValue(org.hl7.fhir.r4.model.CapabilityStatement.ConditionalDeleteStatus.NULL); 045 break; 046 } 047 return tgt; 048 } 049 050 static public org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Conformance.ConditionalDeleteStatus> convertConditionalDeleteStatus(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.CapabilityStatement.ConditionalDeleteStatus> src) throws FHIRException { 051 if (src == null || src.isEmpty()) 052 return null; 053 org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Conformance.ConditionalDeleteStatus> tgt = new org.hl7.fhir.dstu2016may.model.Enumeration<>(new org.hl7.fhir.dstu2016may.model.Conformance.ConditionalDeleteStatusEnumFactory()); 054 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyElement(src, tgt); 055 switch (src.getValue()) { 056 case NOTSUPPORTED: 057 tgt.setValue(org.hl7.fhir.dstu2016may.model.Conformance.ConditionalDeleteStatus.NOTSUPPORTED); 058 break; 059 case SINGLE: 060 tgt.setValue(org.hl7.fhir.dstu2016may.model.Conformance.ConditionalDeleteStatus.SINGLE); 061 break; 062 case MULTIPLE: 063 tgt.setValue(org.hl7.fhir.dstu2016may.model.Conformance.ConditionalDeleteStatus.MULTIPLE); 064 break; 065 default: 066 tgt.setValue(org.hl7.fhir.dstu2016may.model.Conformance.ConditionalDeleteStatus.NULL); 067 break; 068 } 069 return tgt; 070 } 071 072 public static org.hl7.fhir.dstu2016may.model.Conformance convertConformance(org.hl7.fhir.r4.model.CapabilityStatement src) throws FHIRException { 073 if (src == null || src.isEmpty()) 074 return null; 075 org.hl7.fhir.dstu2016may.model.Conformance tgt = new org.hl7.fhir.dstu2016may.model.Conformance(); 076 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyDomainResource(src, tgt, IGNORED_EXTENSION_URLS); 077 if (src.hasUrl()) 078 tgt.setUrlElement(Uri14_40.convertUri(src.getUrlElement())); 079 if (src.hasVersion()) 080 tgt.setVersionElement(String14_40.convertString(src.getVersionElement())); 081 if (src.hasName()) 082 tgt.setNameElement(String14_40.convertString(src.getNameElement())); 083 if (src.hasStatus()) 084 tgt.setStatusElement(Enumerations14_40.convertConformanceResourceStatus(src.getStatusElement())); 085 if (src.hasExperimental()) 086 tgt.setExperimentalElement(Boolean14_40.convertBoolean(src.getExperimentalElement())); 087 if (src.hasDate()) 088 tgt.setDateElement(DateTime14_40.convertDateTime(src.getDateElement())); 089 if (src.hasPublisher()) 090 tgt.setPublisherElement(String14_40.convertString(src.getPublisherElement())); 091 for (org.hl7.fhir.r4.model.ContactDetail t : src.getContact()) 092 tgt.addContact(convertConformanceContactComponent(t)); 093 if (src.hasDescription()) 094 tgt.setDescription(src.getDescription()); 095 for (org.hl7.fhir.r4.model.UsageContext t : src.getUseContext()) 096 if (t.hasValueCodeableConcept()) 097 tgt.addUseContext(CodeableConcept14_40.convertCodeableConcept(t.getValueCodeableConcept())); 098 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getJurisdiction()) 099 tgt.addUseContext(CodeableConcept14_40.convertCodeableConcept(t)); 100 if (src.hasPurpose()) 101 tgt.setRequirements(src.getPurpose()); 102 if (src.hasCopyright()) 103 tgt.setCopyright(src.getCopyright()); 104 if (src.hasKind()) 105 tgt.setKindElement(convertConformanceStatementKind(src.getKindElement())); 106 if (src.hasSoftware()) 107 tgt.setSoftware(convertConformanceSoftwareComponent(src.getSoftware())); 108 if (src.hasImplementation()) 109 tgt.setImplementation(convertConformanceImplementationComponent(src.getImplementation())); 110 tgt.setFhirVersion(src.getFhirVersion().toCode()); 111 if (src.hasExtension(ACCEPT_UNKNOWN_EXTENSION_URL)) 112 tgt.setAcceptUnknown(org.hl7.fhir.dstu2016may.model.Conformance.UnknownContentCode.fromCode(src.getExtensionByUrl(ACCEPT_UNKNOWN_EXTENSION_URL).getValue().primitiveValue())); 113 for (org.hl7.fhir.r4.model.CodeType t : src.getFormat()) tgt.addFormat(t.getValue()); 114 for (CapabilityStatementRestComponent r : src.getRest()) 115 for (CapabilityStatementRestResourceComponent rr : r.getResource()) 116 for (org.hl7.fhir.r4.model.CanonicalType t : rr.getSupportedProfile()) 117 tgt.addProfile(Reference14_40.convertCanonicalToReference(t)); 118 for (org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementRestComponent t : src.getRest()) 119 tgt.addRest(convertConformanceRestComponent(t)); 120 for (org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementMessagingComponent t : src.getMessaging()) 121 tgt.addMessaging(convertConformanceMessagingComponent(t)); 122 for (org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementDocumentComponent t : src.getDocument()) 123 tgt.addDocument(convertConformanceDocumentComponent(t)); 124 return tgt; 125 } 126 127 public static org.hl7.fhir.r4.model.CapabilityStatement convertConformance(org.hl7.fhir.dstu2016may.model.Conformance src) throws FHIRException { 128 if (src == null || src.isEmpty()) 129 return null; 130 org.hl7.fhir.r4.model.CapabilityStatement tgt = new org.hl7.fhir.r4.model.CapabilityStatement(); 131 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyDomainResource(src, tgt); 132 if (src.hasUrl()) 133 tgt.setUrlElement(Uri14_40.convertUri(src.getUrlElement())); 134 if (src.hasVersion()) 135 tgt.setVersionElement(String14_40.convertString(src.getVersionElement())); 136 if (src.hasName()) 137 tgt.setNameElement(String14_40.convertString(src.getNameElement())); 138 if (src.hasStatus()) 139 tgt.setStatusElement(Enumerations14_40.convertConformanceResourceStatus(src.getStatusElement())); 140 if (src.hasExperimental()) 141 tgt.setExperimentalElement(Boolean14_40.convertBoolean(src.getExperimentalElement())); 142 if (src.hasDate()) 143 tgt.setDateElement(DateTime14_40.convertDateTime(src.getDateElement())); 144 if (src.hasPublisher()) 145 tgt.setPublisherElement(String14_40.convertString(src.getPublisherElement())); 146 for (org.hl7.fhir.dstu2016may.model.Conformance.ConformanceContactComponent t : src.getContact()) 147 tgt.addContact(convertConformanceContactComponent(t)); 148 if (src.hasDescription()) 149 tgt.setDescription(src.getDescription()); 150 for (org.hl7.fhir.dstu2016may.model.CodeableConcept t : src.getUseContext()) 151 if (VersionConvertor_14_40.isJurisdiction(t)) 152 tgt.addJurisdiction(CodeableConcept14_40.convertCodeableConcept(t)); 153 else 154 tgt.addUseContext(CodeableConcept14_40.convertCodeableConceptToUsageContext(t)); 155 if (src.hasRequirements()) 156 tgt.setPurpose(src.getRequirements()); 157 if (src.hasCopyright()) 158 tgt.setCopyright(src.getCopyright()); 159 if (src.hasKind()) 160 tgt.setKindElement(convertConformanceStatementKind(src.getKindElement())); 161 if (src.hasSoftware()) 162 tgt.setSoftware(convertConformanceSoftwareComponent(src.getSoftware())); 163 if (src.hasImplementation()) 164 tgt.setImplementation(convertConformanceImplementationComponent(src.getImplementation())); 165 if (src.hasFhirVersion()) 166 tgt.setFhirVersion(org.hl7.fhir.r4.model.Enumerations.FHIRVersion.fromCode(src.getFhirVersion())); 167 if (src.hasAcceptUnknown()) 168 tgt.addExtension().setUrl(ACCEPT_UNKNOWN_EXTENSION_URL).setValue(new org.hl7.fhir.r4.model.CodeType(src.getAcceptUnknownElement().asStringValue())); 169 for (org.hl7.fhir.dstu2016may.model.CodeType t : src.getFormat()) tgt.addFormat(t.getValue()); 170 for (org.hl7.fhir.dstu2016may.model.Conformance.ConformanceRestComponent t : src.getRest()) 171 tgt.addRest(convertConformanceRestComponent(t)); 172 for (org.hl7.fhir.dstu2016may.model.Conformance.ConformanceMessagingComponent t : src.getMessaging()) 173 tgt.addMessaging(convertConformanceMessagingComponent(t)); 174 for (org.hl7.fhir.dstu2016may.model.Conformance.ConformanceDocumentComponent t : src.getDocument()) 175 tgt.addDocument(convertConformanceDocumentComponent(t)); 176 return tgt; 177 } 178 179 public static org.hl7.fhir.dstu2016may.model.Conformance.ConformanceContactComponent convertConformanceContactComponent(org.hl7.fhir.r4.model.ContactDetail src) throws FHIRException { 180 if (src == null || src.isEmpty()) 181 return null; 182 org.hl7.fhir.dstu2016may.model.Conformance.ConformanceContactComponent tgt = new org.hl7.fhir.dstu2016may.model.Conformance.ConformanceContactComponent(); 183 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyElement(src, tgt); 184 if (src.hasName()) 185 tgt.setNameElement(String14_40.convertString(src.getNameElement())); 186 for (org.hl7.fhir.r4.model.ContactPoint t : src.getTelecom()) 187 tgt.addTelecom(ContactPoint14_40.convertContactPoint(t)); 188 return tgt; 189 } 190 191 public static org.hl7.fhir.r4.model.ContactDetail convertConformanceContactComponent(org.hl7.fhir.dstu2016may.model.Conformance.ConformanceContactComponent src) throws FHIRException { 192 if (src == null || src.isEmpty()) 193 return null; 194 org.hl7.fhir.r4.model.ContactDetail tgt = new org.hl7.fhir.r4.model.ContactDetail(); 195 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyElement(src, tgt); 196 if (src.hasName()) 197 tgt.setNameElement(String14_40.convertString(src.getNameElement())); 198 for (org.hl7.fhir.dstu2016may.model.ContactPoint t : src.getTelecom()) 199 tgt.addTelecom(ContactPoint14_40.convertContactPoint(t)); 200 return tgt; 201 } 202 203 public static org.hl7.fhir.dstu2016may.model.Conformance.ConformanceDocumentComponent convertConformanceDocumentComponent(org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementDocumentComponent src) throws FHIRException { 204 if (src == null || src.isEmpty()) 205 return null; 206 org.hl7.fhir.dstu2016may.model.Conformance.ConformanceDocumentComponent tgt = new org.hl7.fhir.dstu2016may.model.Conformance.ConformanceDocumentComponent(); 207 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyBackboneElement(src,tgt); 208 if (src.hasMode()) 209 tgt.setModeElement(convertDocumentMode(src.getModeElement())); 210 if (src.hasDocumentation()) 211 tgt.setDocumentation(src.getDocumentation()); 212 if (src.hasProfileElement()) 213 tgt.setProfile(Reference14_40.convertCanonicalToReference(src.getProfileElement())); 214 return tgt; 215 } 216 217 public static org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementDocumentComponent convertConformanceDocumentComponent(org.hl7.fhir.dstu2016may.model.Conformance.ConformanceDocumentComponent src) throws FHIRException { 218 if (src == null || src.isEmpty()) 219 return null; 220 org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementDocumentComponent tgt = new org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementDocumentComponent(); 221 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyBackboneElement(src,tgt); 222 if (src.hasMode()) 223 tgt.setModeElement(convertDocumentMode(src.getModeElement())); 224 if (src.hasDocumentation()) 225 tgt.setDocumentation(src.getDocumentation()); 226 if (src.hasProfile()) 227 tgt.setProfileElement(Reference14_40.convertReferenceToCanonical(src.getProfile())); 228 return tgt; 229 } 230 231 public static org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementImplementationComponent convertConformanceImplementationComponent(org.hl7.fhir.dstu2016may.model.Conformance.ConformanceImplementationComponent src) throws FHIRException { 232 if (src == null || src.isEmpty()) 233 return null; 234 org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementImplementationComponent tgt = new org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementImplementationComponent(); 235 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyBackboneElement(src,tgt); 236 if (src.hasDescriptionElement()) 237 tgt.setDescriptionElement(String14_40.convertString(src.getDescriptionElement())); 238 if (src.hasUrl()) 239 tgt.setUrl(src.getUrl()); 240 return tgt; 241 } 242 243 public static org.hl7.fhir.dstu2016may.model.Conformance.ConformanceImplementationComponent convertConformanceImplementationComponent(org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementImplementationComponent src) throws FHIRException { 244 if (src == null || src.isEmpty()) 245 return null; 246 org.hl7.fhir.dstu2016may.model.Conformance.ConformanceImplementationComponent tgt = new org.hl7.fhir.dstu2016may.model.Conformance.ConformanceImplementationComponent(); 247 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyBackboneElement(src,tgt); 248 if (src.hasDescriptionElement()) 249 tgt.setDescriptionElement(String14_40.convertString(src.getDescriptionElement())); 250 if (src.hasUrl()) 251 tgt.setUrl(src.getUrl()); 252 return tgt; 253 } 254 255 public static org.hl7.fhir.dstu2016may.model.Conformance.ConformanceMessagingComponent convertConformanceMessagingComponent(org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementMessagingComponent src) throws FHIRException { 256 if (src == null || src.isEmpty()) 257 return null; 258 org.hl7.fhir.dstu2016may.model.Conformance.ConformanceMessagingComponent tgt = new org.hl7.fhir.dstu2016may.model.Conformance.ConformanceMessagingComponent(); 259 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyBackboneElement(src,tgt); 260 for (org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementMessagingEndpointComponent t : src.getEndpoint()) 261 tgt.addEndpoint(convertConformanceMessagingEndpointComponent(t)); 262 if (src.hasReliableCache()) 263 tgt.setReliableCacheElement(UnsignedInt14_40.convertUnsignedInt(src.getReliableCacheElement())); 264 if (src.hasDocumentation()) 265 tgt.setDocumentation(src.getDocumentation()); 266 for (org.hl7.fhir.r4.model.Extension e : src.getExtensionsByUrl(VersionConvertorConstants.IG_CONFORMANCE_MESSAGE_EVENT)) { 267 org.hl7.fhir.dstu2016may.model.Conformance.ConformanceMessagingEventComponent event = new org.hl7.fhir.dstu2016may.model.Conformance.ConformanceMessagingEventComponent(); 268 tgt.addEvent(event); 269 event.setCode(Coding14_40.convertCoding((org.hl7.fhir.r4.model.Coding) e.getExtensionByUrl("code").getValue())); 270 if (e.hasExtension("category")) 271 event.setCategory(org.hl7.fhir.dstu2016may.model.Conformance.MessageSignificanceCategory.fromCode(e.getExtensionByUrl("category").getValue().toString())); 272 event.setMode(org.hl7.fhir.dstu2016may.model.Conformance.ConformanceEventMode.fromCode(e.getExtensionByUrl("mode").getValue().toString())); 273 event.setCode(Coding14_40.convertCoding((org.hl7.fhir.r4.model.Coding) e.getExtensionByUrl("code").getValue())); 274 if (e.hasExtension("category")) 275 event.setCategory(org.hl7.fhir.dstu2016may.model.Conformance.MessageSignificanceCategory.fromCode(e.getExtensionByUrl("category").getValue().toString())); 276 event.setMode(org.hl7.fhir.dstu2016may.model.Conformance.ConformanceEventMode.fromCode(e.getExtensionByUrl("mode").getValue().toString())); 277 org.hl7.fhir.r4.model.Extension focusE = e.getExtensionByUrl("focus"); 278 if (focusE.getValue().hasPrimitiveValue()) 279 event.setFocus(focusE.getValue().toString()); 280 else { 281 event.setFocusElement(new org.hl7.fhir.dstu2016may.model.CodeType()); 282 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyElement(focusE.getValue(), event.getFocusElement()); 283 } 284 event.setRequest(Reference14_40.convertReference((org.hl7.fhir.r4.model.Reference) e.getExtensionByUrl("request").getValue())); 285 event.setResponse(Reference14_40.convertReference((org.hl7.fhir.r4.model.Reference) e.getExtensionByUrl("response").getValue())); 286 if (e.hasExtension("documentation")) 287 event.setDocumentation(e.getExtensionByUrl("documentation").getValue().toString()); 288 } 289 return tgt; 290 } 291 292 public static org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementMessagingComponent convertConformanceMessagingComponent(org.hl7.fhir.dstu2016may.model.Conformance.ConformanceMessagingComponent src) throws FHIRException { 293 if (src == null || src.isEmpty()) 294 return null; 295 org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementMessagingComponent tgt = new org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementMessagingComponent(); 296 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyBackboneElement(src,tgt); 297 for (org.hl7.fhir.dstu2016may.model.Conformance.ConformanceMessagingEndpointComponent t : src.getEndpoint()) 298 tgt.addEndpoint(convertConformanceMessagingEndpointComponent(t)); 299 if (src.hasReliableCache()) 300 tgt.setReliableCacheElement(UnsignedInt14_40.convertUnsignedInt(src.getReliableCacheElement())); 301 if (src.hasDocumentation()) 302 tgt.setDocumentation(src.getDocumentation()); 303 for (org.hl7.fhir.dstu2016may.model.Conformance.ConformanceMessagingEventComponent t : src.getEvent()) { 304 org.hl7.fhir.r4.model.Extension e = new org.hl7.fhir.r4.model.Extension(VersionConvertorConstants.IG_CONFORMANCE_MESSAGE_EVENT); 305 e.addExtension(new org.hl7.fhir.r4.model.Extension("code", Coding14_40.convertCoding(t.getCode()))); 306 if (t.hasCategory()) 307 e.addExtension(new org.hl7.fhir.r4.model.Extension("category", new org.hl7.fhir.r4.model.CodeType(t.getCategory().toCode()))); 308 e.addExtension(new org.hl7.fhir.r4.model.Extension("mode", new org.hl7.fhir.r4.model.CodeType(t.getMode().toCode()))); 309 if (t.getFocusElement().hasValue()) 310 e.addExtension(new org.hl7.fhir.r4.model.Extension("focus", new org.hl7.fhir.r4.model.StringType(t.getFocus()))); 311 else { 312 org.hl7.fhir.r4.model.CodeType focus = new org.hl7.fhir.r4.model.CodeType(); 313 org.hl7.fhir.r4.model.Extension focusE = new org.hl7.fhir.r4.model.Extension("focus", focus); 314 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyElement(t.getFocusElement(), focus); 315 e.addExtension(focusE); 316 } 317 e.addExtension(new org.hl7.fhir.r4.model.Extension("request", Reference14_40.convertReference(t.getRequest()))); 318 e.addExtension(new org.hl7.fhir.r4.model.Extension("response", Reference14_40.convertReference(t.getResponse()))); 319 if (t.hasDocumentation()) 320 e.addExtension(new org.hl7.fhir.r4.model.Extension("documentation", new org.hl7.fhir.r4.model.StringType(t.getDocumentation()))); 321 tgt.addExtension(e); 322 } 323 return tgt; 324 } 325 326 public static org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementMessagingEndpointComponent convertConformanceMessagingEndpointComponent(org.hl7.fhir.dstu2016may.model.Conformance.ConformanceMessagingEndpointComponent src) throws FHIRException { 327 if (src == null || src.isEmpty()) 328 return null; 329 org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementMessagingEndpointComponent tgt = new org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementMessagingEndpointComponent(); 330 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyBackboneElement(src,tgt); 331 if (src.hasProtocol()) 332 tgt.setProtocol(Coding14_40.convertCoding(src.getProtocol())); 333 if (src.hasAddress()) 334 tgt.setAddress(src.getAddress()); 335 return tgt; 336 } 337 338 public static org.hl7.fhir.dstu2016may.model.Conformance.ConformanceMessagingEndpointComponent convertConformanceMessagingEndpointComponent(org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementMessagingEndpointComponent src) throws FHIRException { 339 if (src == null || src.isEmpty()) 340 return null; 341 org.hl7.fhir.dstu2016may.model.Conformance.ConformanceMessagingEndpointComponent tgt = new org.hl7.fhir.dstu2016may.model.Conformance.ConformanceMessagingEndpointComponent(); 342 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyBackboneElement(src,tgt); 343 if (src.hasProtocol()) 344 tgt.setProtocol(Coding14_40.convertCoding(src.getProtocol())); 345 if (src.hasAddress()) 346 tgt.setAddress(src.getAddress()); 347 return tgt; 348 } 349 350 public static org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementRestComponent convertConformanceRestComponent(org.hl7.fhir.dstu2016may.model.Conformance.ConformanceRestComponent src) throws FHIRException { 351 if (src == null || src.isEmpty()) 352 return null; 353 org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementRestComponent tgt = new org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementRestComponent(); 354 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyBackboneElement(src,tgt); 355 if (src.hasMode()) 356 tgt.setModeElement(convertRestfulConformanceMode(src.getModeElement())); 357 if (src.hasDocumentation()) 358 tgt.setDocumentation(src.getDocumentation()); 359 if (src.hasSecurity()) 360 tgt.setSecurity(convertConformanceRestSecurityComponent(src.getSecurity())); 361 for (org.hl7.fhir.dstu2016may.model.Conformance.ConformanceRestResourceComponent t : src.getResource()) 362 tgt.addResource(convertConformanceRestResourceComponent(t)); 363 for (org.hl7.fhir.dstu2016may.model.Conformance.SystemInteractionComponent t : src.getInteraction()) 364 tgt.addInteraction(convertSystemInteractionComponent(t)); 365 for (org.hl7.fhir.dstu2016may.model.Conformance.ConformanceRestResourceSearchParamComponent t : src.getSearchParam()) 366 tgt.addSearchParam(convertConformanceRestResourceSearchParamComponent(t)); 367 for (org.hl7.fhir.dstu2016may.model.Conformance.ConformanceRestOperationComponent t : src.getOperation()) 368 tgt.addOperation(convertConformanceRestOperationComponent(t)); 369 for (org.hl7.fhir.dstu2016may.model.UriType t : src.getCompartment()) tgt.addCompartment(t.getValue()); 370 return tgt; 371 } 372 373 public static org.hl7.fhir.dstu2016may.model.Conformance.ConformanceRestComponent convertConformanceRestComponent(org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementRestComponent src) throws FHIRException { 374 if (src == null || src.isEmpty()) 375 return null; 376 org.hl7.fhir.dstu2016may.model.Conformance.ConformanceRestComponent tgt = new org.hl7.fhir.dstu2016may.model.Conformance.ConformanceRestComponent(); 377 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyBackboneElement(src,tgt); 378 if (src.hasMode()) 379 tgt.setModeElement(convertRestfulConformanceMode(src.getModeElement())); 380 if (src.hasDocumentation()) 381 tgt.setDocumentation(src.getDocumentation()); 382 if (src.hasSecurity()) 383 tgt.setSecurity(convertConformanceRestSecurityComponent(src.getSecurity())); 384 for (org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementRestResourceComponent t : src.getResource()) 385 tgt.addResource(convertConformanceRestResourceComponent(t)); 386 for (org.hl7.fhir.r4.model.CapabilityStatement.SystemInteractionComponent t : src.getInteraction()) 387 tgt.addInteraction(convertSystemInteractionComponent(t)); 388 for (org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementRestResourceSearchParamComponent t : src.getSearchParam()) 389 tgt.addSearchParam(convertConformanceRestResourceSearchParamComponent(t)); 390 for (org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementRestResourceOperationComponent t : src.getOperation()) 391 tgt.addOperation(convertConformanceRestOperationComponent(t)); 392 for (org.hl7.fhir.r4.model.UriType t : src.getCompartment()) tgt.addCompartment(t.getValue()); 393 return tgt; 394 } 395 396 public static org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementRestResourceOperationComponent convertConformanceRestOperationComponent(org.hl7.fhir.dstu2016may.model.Conformance.ConformanceRestOperationComponent src) throws FHIRException { 397 if (src == null || src.isEmpty()) 398 return null; 399 org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementRestResourceOperationComponent tgt = new org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementRestResourceOperationComponent(); 400 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyBackboneElement(src,tgt); 401 if (src.hasNameElement()) 402 tgt.setNameElement(String14_40.convertString(src.getNameElement())); 403 if (src.hasDefinition()) 404 tgt.setDefinitionElement(Reference14_40.convertReferenceToCanonical(src.getDefinition())); 405 return tgt; 406 } 407 408 public static org.hl7.fhir.dstu2016may.model.Conformance.ConformanceRestOperationComponent convertConformanceRestOperationComponent(org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementRestResourceOperationComponent src) throws FHIRException { 409 if (src == null || src.isEmpty()) 410 return null; 411 org.hl7.fhir.dstu2016may.model.Conformance.ConformanceRestOperationComponent tgt = new org.hl7.fhir.dstu2016may.model.Conformance.ConformanceRestOperationComponent(); 412 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyBackboneElement(src,tgt); 413 if (src.hasNameElement()) 414 tgt.setNameElement(String14_40.convertString(src.getNameElement())); 415 if (src.hasDefinitionElement()) 416 tgt.setDefinition(Reference14_40.convertCanonicalToReference(src.getDefinitionElement())); 417 return tgt; 418 } 419 420 public static org.hl7.fhir.dstu2016may.model.Conformance.ConformanceRestResourceComponent convertConformanceRestResourceComponent(org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementRestResourceComponent src) throws FHIRException { 421 if (src == null || src.isEmpty()) 422 return null; 423 org.hl7.fhir.dstu2016may.model.Conformance.ConformanceRestResourceComponent tgt = new org.hl7.fhir.dstu2016may.model.Conformance.ConformanceRestResourceComponent(); 424 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyBackboneElement(src,tgt); 425 if (src.hasTypeElement()) 426 tgt.setTypeElement(Code14_40.convertCode(src.getTypeElement())); 427 if (src.hasProfileElement()) 428 tgt.setProfile(Reference14_40.convertCanonicalToReference(src.getProfileElement())); 429 for (org.hl7.fhir.r4.model.CapabilityStatement.ResourceInteractionComponent t : src.getInteraction()) 430 tgt.addInteraction(convertResourceInteractionComponent(t)); 431 if (src.hasVersioning()) 432 tgt.setVersioningElement(convertResourceVersionPolicy(src.getVersioningElement())); 433 if (src.hasReadHistory()) 434 tgt.setReadHistoryElement(Boolean14_40.convertBoolean(src.getReadHistoryElement())); 435 if (src.hasUpdateCreate()) 436 tgt.setUpdateCreateElement(Boolean14_40.convertBoolean(src.getUpdateCreateElement())); 437 if (src.hasConditionalCreate()) 438 tgt.setConditionalCreateElement(Boolean14_40.convertBoolean(src.getConditionalCreateElement())); 439 if (src.hasConditionalUpdate()) 440 tgt.setConditionalUpdateElement(Boolean14_40.convertBoolean(src.getConditionalUpdateElement())); 441 if (src.hasConditionalDelete()) 442 tgt.setConditionalDeleteElement(convertConditionalDeleteStatus(src.getConditionalDeleteElement())); 443 for (org.hl7.fhir.r4.model.StringType t : src.getSearchInclude()) tgt.addSearchInclude(t.getValue()); 444 for (org.hl7.fhir.r4.model.StringType t : src.getSearchRevInclude()) tgt.addSearchRevInclude(t.getValue()); 445 for (org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementRestResourceSearchParamComponent t : src.getSearchParam()) 446 tgt.addSearchParam(convertConformanceRestResourceSearchParamComponent(t)); 447 return tgt; 448 } 449 450 public static org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementRestResourceComponent convertConformanceRestResourceComponent(org.hl7.fhir.dstu2016may.model.Conformance.ConformanceRestResourceComponent src) throws FHIRException { 451 if (src == null || src.isEmpty()) 452 return null; 453 org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementRestResourceComponent tgt = new org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementRestResourceComponent(); 454 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyBackboneElement(src,tgt); 455 if (src.hasTypeElement()) 456 tgt.setTypeElement(Code14_40.convertCode(src.getTypeElement())); 457 if (src.hasProfile()) 458 tgt.setProfileElement(Reference14_40.convertReferenceToCanonical(src.getProfile())); 459 for (org.hl7.fhir.dstu2016may.model.Conformance.ResourceInteractionComponent t : src.getInteraction()) 460 tgt.addInteraction(convertResourceInteractionComponent(t)); 461 if (src.hasVersioning()) 462 tgt.setVersioningElement(convertResourceVersionPolicy(src.getVersioningElement())); 463 if (src.hasReadHistory()) 464 tgt.setReadHistoryElement(Boolean14_40.convertBoolean(src.getReadHistoryElement())); 465 if (src.hasUpdateCreate()) 466 tgt.setUpdateCreateElement(Boolean14_40.convertBoolean(src.getUpdateCreateElement())); 467 if (src.hasConditionalCreate()) 468 tgt.setConditionalCreateElement(Boolean14_40.convertBoolean(src.getConditionalCreateElement())); 469 if (src.hasConditionalUpdate()) 470 tgt.setConditionalUpdateElement(Boolean14_40.convertBoolean(src.getConditionalUpdateElement())); 471 if (src.hasConditionalDelete()) 472 tgt.setConditionalDeleteElement(convertConditionalDeleteStatus(src.getConditionalDeleteElement())); 473 for (org.hl7.fhir.dstu2016may.model.StringType t : src.getSearchInclude()) tgt.addSearchInclude(t.getValue()); 474 for (org.hl7.fhir.dstu2016may.model.StringType t : src.getSearchRevInclude()) tgt.addSearchRevInclude(t.getValue()); 475 for (org.hl7.fhir.dstu2016may.model.Conformance.ConformanceRestResourceSearchParamComponent t : src.getSearchParam()) 476 tgt.addSearchParam(convertConformanceRestResourceSearchParamComponent(t)); 477 return tgt; 478 } 479 480 public static org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementRestResourceSearchParamComponent convertConformanceRestResourceSearchParamComponent(org.hl7.fhir.dstu2016may.model.Conformance.ConformanceRestResourceSearchParamComponent src) throws FHIRException { 481 if (src == null || src.isEmpty()) 482 return null; 483 org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementRestResourceSearchParamComponent tgt = new org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementRestResourceSearchParamComponent(); 484 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyBackboneElement(src,tgt); 485 if (src.hasNameElement()) 486 tgt.setNameElement(String14_40.convertString(src.getNameElement())); 487 if (src.hasDefinition()) 488 tgt.setDefinition(src.getDefinition()); 489 if (src.hasType()) 490 tgt.setTypeElement(Enumerations14_40.convertSearchParamType(src.getTypeElement())); 491 if (src.hasDocumentation()) 492 tgt.setDocumentation(src.getDocumentation()); 493 return tgt; 494 } 495 496 public static org.hl7.fhir.dstu2016may.model.Conformance.ConformanceRestResourceSearchParamComponent convertConformanceRestResourceSearchParamComponent(org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementRestResourceSearchParamComponent src) throws FHIRException { 497 if (src == null || src.isEmpty()) 498 return null; 499 org.hl7.fhir.dstu2016may.model.Conformance.ConformanceRestResourceSearchParamComponent tgt = new org.hl7.fhir.dstu2016may.model.Conformance.ConformanceRestResourceSearchParamComponent(); 500 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyBackboneElement(src,tgt); 501 if (src.hasNameElement()) 502 tgt.setNameElement(String14_40.convertString(src.getNameElement())); 503 if (src.hasDefinition()) 504 tgt.setDefinition(src.getDefinition()); 505 if (src.hasType()) 506 tgt.setTypeElement(Enumerations14_40.convertSearchParamType(src.getTypeElement())); 507 if (src.hasDocumentation()) 508 tgt.setDocumentation(src.getDocumentation()); 509 return tgt; 510 } 511 512 public static org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementRestSecurityComponent convertConformanceRestSecurityComponent(org.hl7.fhir.dstu2016may.model.Conformance.ConformanceRestSecurityComponent src) throws FHIRException { 513 if (src == null || src.isEmpty()) 514 return null; 515 org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementRestSecurityComponent tgt = new org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementRestSecurityComponent(); 516 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyBackboneElement(src,tgt); 517 if (src.hasCors()) 518 tgt.setCorsElement(Boolean14_40.convertBoolean(src.getCorsElement())); 519 for (org.hl7.fhir.dstu2016may.model.CodeableConcept t : src.getService()) 520 tgt.addService(CodeableConcept14_40.convertCodeableConcept(t)); 521 if (src.hasDescription()) 522 tgt.setDescription(src.getDescription()); 523 return tgt; 524 } 525 526 public static org.hl7.fhir.dstu2016may.model.Conformance.ConformanceRestSecurityComponent convertConformanceRestSecurityComponent(org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementRestSecurityComponent src) throws FHIRException { 527 if (src == null || src.isEmpty()) 528 return null; 529 org.hl7.fhir.dstu2016may.model.Conformance.ConformanceRestSecurityComponent tgt = new org.hl7.fhir.dstu2016may.model.Conformance.ConformanceRestSecurityComponent(); 530 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyBackboneElement(src,tgt); 531 if (src.hasCors()) 532 tgt.setCorsElement(Boolean14_40.convertBoolean(src.getCorsElement())); 533 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getService()) 534 tgt.addService(CodeableConcept14_40.convertCodeableConcept(t)); 535 if (src.hasDescription()) 536 tgt.setDescription(src.getDescription()); 537 return tgt; 538 } 539 540 public static org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementSoftwareComponent convertConformanceSoftwareComponent(org.hl7.fhir.dstu2016may.model.Conformance.ConformanceSoftwareComponent src) throws FHIRException { 541 if (src == null || src.isEmpty()) 542 return null; 543 org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementSoftwareComponent tgt = new org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementSoftwareComponent(); 544 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyBackboneElement(src,tgt); 545 if (src.hasNameElement()) 546 tgt.setNameElement(String14_40.convertString(src.getNameElement())); 547 if (src.hasVersion()) 548 tgt.setVersionElement(String14_40.convertString(src.getVersionElement())); 549 if (src.hasReleaseDate()) 550 tgt.setReleaseDateElement(DateTime14_40.convertDateTime(src.getReleaseDateElement())); 551 return tgt; 552 } 553 554 public static org.hl7.fhir.dstu2016may.model.Conformance.ConformanceSoftwareComponent convertConformanceSoftwareComponent(org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementSoftwareComponent src) throws FHIRException { 555 if (src == null || src.isEmpty()) 556 return null; 557 org.hl7.fhir.dstu2016may.model.Conformance.ConformanceSoftwareComponent tgt = new org.hl7.fhir.dstu2016may.model.Conformance.ConformanceSoftwareComponent(); 558 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyBackboneElement(src,tgt); 559 if (src.hasNameElement()) 560 tgt.setNameElement(String14_40.convertString(src.getNameElement())); 561 if (src.hasVersion()) 562 tgt.setVersionElement(String14_40.convertString(src.getVersionElement())); 563 if (src.hasReleaseDate()) 564 tgt.setReleaseDateElement(DateTime14_40.convertDateTime(src.getReleaseDateElement())); 565 return tgt; 566 } 567 568 static public org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Conformance.ConformanceStatementKind> convertConformanceStatementKind(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementKind> src) throws FHIRException { 569 if (src == null || src.isEmpty()) 570 return null; 571 org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Conformance.ConformanceStatementKind> tgt = new org.hl7.fhir.dstu2016may.model.Enumeration<>(new org.hl7.fhir.dstu2016may.model.Conformance.ConformanceStatementKindEnumFactory()); 572 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyElement(src, tgt); 573 switch (src.getValue()) { 574 case INSTANCE: 575 tgt.setValue(org.hl7.fhir.dstu2016may.model.Conformance.ConformanceStatementKind.INSTANCE); 576 break; 577 case CAPABILITY: 578 tgt.setValue(org.hl7.fhir.dstu2016may.model.Conformance.ConformanceStatementKind.CAPABILITY); 579 break; 580 case REQUIREMENTS: 581 tgt.setValue(org.hl7.fhir.dstu2016may.model.Conformance.ConformanceStatementKind.REQUIREMENTS); 582 break; 583 default: 584 tgt.setValue(org.hl7.fhir.dstu2016may.model.Conformance.ConformanceStatementKind.NULL); 585 break; 586 } 587 return tgt; 588 } 589 590 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementKind> convertConformanceStatementKind(org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Conformance.ConformanceStatementKind> src) throws FHIRException { 591 if (src == null || src.isEmpty()) 592 return null; 593 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementKind> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementKindEnumFactory()); 594 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyElement(src, tgt); 595 switch (src.getValue()) { 596 case INSTANCE: 597 tgt.setValue(org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementKind.INSTANCE); 598 break; 599 case CAPABILITY: 600 tgt.setValue(org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementKind.CAPABILITY); 601 break; 602 case REQUIREMENTS: 603 tgt.setValue(org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementKind.REQUIREMENTS); 604 break; 605 default: 606 tgt.setValue(org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementKind.NULL); 607 break; 608 } 609 return tgt; 610 } 611 612 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.CapabilityStatement.DocumentMode> convertDocumentMode(org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Conformance.DocumentMode> src) throws FHIRException { 613 if (src == null || src.isEmpty()) 614 return null; 615 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.CapabilityStatement.DocumentMode> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.CapabilityStatement.DocumentModeEnumFactory()); 616 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyElement(src, tgt); 617 switch (src.getValue()) { 618 case PRODUCER: 619 tgt.setValue(org.hl7.fhir.r4.model.CapabilityStatement.DocumentMode.PRODUCER); 620 break; 621 case CONSUMER: 622 tgt.setValue(org.hl7.fhir.r4.model.CapabilityStatement.DocumentMode.CONSUMER); 623 break; 624 default: 625 tgt.setValue(org.hl7.fhir.r4.model.CapabilityStatement.DocumentMode.NULL); 626 break; 627 } 628 return tgt; 629 } 630 631 static public org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Conformance.DocumentMode> convertDocumentMode(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.CapabilityStatement.DocumentMode> src) throws FHIRException { 632 if (src == null || src.isEmpty()) 633 return null; 634 org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Conformance.DocumentMode> tgt = new org.hl7.fhir.dstu2016may.model.Enumeration<>(new org.hl7.fhir.dstu2016may.model.Conformance.DocumentModeEnumFactory()); 635 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyElement(src, tgt); 636 switch (src.getValue()) { 637 case PRODUCER: 638 tgt.setValue(org.hl7.fhir.dstu2016may.model.Conformance.DocumentMode.PRODUCER); 639 break; 640 case CONSUMER: 641 tgt.setValue(org.hl7.fhir.dstu2016may.model.Conformance.DocumentMode.CONSUMER); 642 break; 643 default: 644 tgt.setValue(org.hl7.fhir.dstu2016may.model.Conformance.DocumentMode.NULL); 645 break; 646 } 647 return tgt; 648 } 649 650 public static org.hl7.fhir.r4.model.CapabilityStatement.ResourceInteractionComponent convertResourceInteractionComponent(org.hl7.fhir.dstu2016may.model.Conformance.ResourceInteractionComponent src) throws FHIRException { 651 if (src == null || src.isEmpty()) 652 return null; 653 org.hl7.fhir.r4.model.CapabilityStatement.ResourceInteractionComponent tgt = new org.hl7.fhir.r4.model.CapabilityStatement.ResourceInteractionComponent(); 654 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyBackboneElement(src,tgt); 655 if (src.hasCode()) 656 tgt.setCodeElement(convertTypeRestfulInteraction(src.getCodeElement())); 657 if (src.hasDocumentation()) 658 tgt.setDocumentation(src.getDocumentation()); 659 return tgt; 660 } 661 662 public static org.hl7.fhir.dstu2016may.model.Conformance.ResourceInteractionComponent convertResourceInteractionComponent(org.hl7.fhir.r4.model.CapabilityStatement.ResourceInteractionComponent src) throws FHIRException { 663 if (src == null || src.isEmpty()) 664 return null; 665 org.hl7.fhir.dstu2016may.model.Conformance.ResourceInteractionComponent tgt = new org.hl7.fhir.dstu2016may.model.Conformance.ResourceInteractionComponent(); 666 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyBackboneElement(src,tgt); 667 if (src.hasCode()) 668 tgt.setCodeElement(convertTypeRestfulInteraction(src.getCodeElement())); 669 if (src.hasDocumentation()) 670 tgt.setDocumentation(src.getDocumentation()); 671 return tgt; 672 } 673 674 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.CapabilityStatement.ResourceVersionPolicy> convertResourceVersionPolicy(org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Conformance.ResourceVersionPolicy> src) throws FHIRException { 675 if (src == null || src.isEmpty()) 676 return null; 677 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.CapabilityStatement.ResourceVersionPolicy> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.CapabilityStatement.ResourceVersionPolicyEnumFactory()); 678 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyElement(src, tgt); 679 switch (src.getValue()) { 680 case NOVERSION: 681 tgt.setValue(org.hl7.fhir.r4.model.CapabilityStatement.ResourceVersionPolicy.NOVERSION); 682 break; 683 case VERSIONED: 684 tgt.setValue(org.hl7.fhir.r4.model.CapabilityStatement.ResourceVersionPolicy.VERSIONED); 685 break; 686 case VERSIONEDUPDATE: 687 tgt.setValue(org.hl7.fhir.r4.model.CapabilityStatement.ResourceVersionPolicy.VERSIONEDUPDATE); 688 break; 689 default: 690 tgt.setValue(org.hl7.fhir.r4.model.CapabilityStatement.ResourceVersionPolicy.NULL); 691 break; 692 } 693 return tgt; 694 } 695 696 static public org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Conformance.ResourceVersionPolicy> convertResourceVersionPolicy(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.CapabilityStatement.ResourceVersionPolicy> src) throws FHIRException { 697 if (src == null || src.isEmpty()) 698 return null; 699 org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Conformance.ResourceVersionPolicy> tgt = new org.hl7.fhir.dstu2016may.model.Enumeration<>(new org.hl7.fhir.dstu2016may.model.Conformance.ResourceVersionPolicyEnumFactory()); 700 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyElement(src, tgt); 701 switch (src.getValue()) { 702 case NOVERSION: 703 tgt.setValue(org.hl7.fhir.dstu2016may.model.Conformance.ResourceVersionPolicy.NOVERSION); 704 break; 705 case VERSIONED: 706 tgt.setValue(org.hl7.fhir.dstu2016may.model.Conformance.ResourceVersionPolicy.VERSIONED); 707 break; 708 case VERSIONEDUPDATE: 709 tgt.setValue(org.hl7.fhir.dstu2016may.model.Conformance.ResourceVersionPolicy.VERSIONEDUPDATE); 710 break; 711 default: 712 tgt.setValue(org.hl7.fhir.dstu2016may.model.Conformance.ResourceVersionPolicy.NULL); 713 break; 714 } 715 return tgt; 716 } 717 718 static public org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Conformance.RestfulConformanceMode> convertRestfulConformanceMode(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.CapabilityStatement.RestfulCapabilityMode> src) throws FHIRException { 719 if (src == null || src.isEmpty()) 720 return null; 721 org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Conformance.RestfulConformanceMode> tgt = new org.hl7.fhir.dstu2016may.model.Enumeration<>(new org.hl7.fhir.dstu2016may.model.Conformance.RestfulConformanceModeEnumFactory()); 722 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyElement(src, tgt); 723 switch (src.getValue()) { 724 case CLIENT: 725 tgt.setValue(org.hl7.fhir.dstu2016may.model.Conformance.RestfulConformanceMode.CLIENT); 726 break; 727 case SERVER: 728 tgt.setValue(org.hl7.fhir.dstu2016may.model.Conformance.RestfulConformanceMode.SERVER); 729 break; 730 default: 731 tgt.setValue(org.hl7.fhir.dstu2016may.model.Conformance.RestfulConformanceMode.NULL); 732 break; 733 } 734 return tgt; 735 } 736 737 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.CapabilityStatement.RestfulCapabilityMode> convertRestfulConformanceMode(org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Conformance.RestfulConformanceMode> src) throws FHIRException { 738 if (src == null || src.isEmpty()) 739 return null; 740 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.CapabilityStatement.RestfulCapabilityMode> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.CapabilityStatement.RestfulCapabilityModeEnumFactory()); 741 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyElement(src, tgt); 742 switch (src.getValue()) { 743 case CLIENT: 744 tgt.setValue(org.hl7.fhir.r4.model.CapabilityStatement.RestfulCapabilityMode.CLIENT); 745 break; 746 case SERVER: 747 tgt.setValue(org.hl7.fhir.r4.model.CapabilityStatement.RestfulCapabilityMode.SERVER); 748 break; 749 default: 750 tgt.setValue(org.hl7.fhir.r4.model.CapabilityStatement.RestfulCapabilityMode.NULL); 751 break; 752 } 753 return tgt; 754 } 755 756 public static org.hl7.fhir.dstu2016may.model.Conformance.SystemInteractionComponent convertSystemInteractionComponent(org.hl7.fhir.r4.model.CapabilityStatement.SystemInteractionComponent src) throws FHIRException { 757 if (src == null || src.isEmpty()) 758 return null; 759 org.hl7.fhir.dstu2016may.model.Conformance.SystemInteractionComponent tgt = new org.hl7.fhir.dstu2016may.model.Conformance.SystemInteractionComponent(); 760 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyBackboneElement(src,tgt); 761 if (src.hasCode()) 762 tgt.setCodeElement(convertSystemRestfulInteraction(src.getCodeElement())); 763 if (src.hasDocumentation()) 764 tgt.setDocumentation(src.getDocumentation()); 765 return tgt; 766 } 767 768 public static org.hl7.fhir.r4.model.CapabilityStatement.SystemInteractionComponent convertSystemInteractionComponent(org.hl7.fhir.dstu2016may.model.Conformance.SystemInteractionComponent src) throws FHIRException { 769 if (src == null || src.isEmpty()) 770 return null; 771 org.hl7.fhir.r4.model.CapabilityStatement.SystemInteractionComponent tgt = new org.hl7.fhir.r4.model.CapabilityStatement.SystemInteractionComponent(); 772 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyBackboneElement(src,tgt); 773 if (src.hasCode()) 774 tgt.setCodeElement(convertSystemRestfulInteraction(src.getCodeElement())); 775 if (src.hasDocumentation()) 776 tgt.setDocumentation(src.getDocumentation()); 777 return tgt; 778 } 779 780 static public org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Conformance.SystemRestfulInteraction> convertSystemRestfulInteraction(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.CapabilityStatement.SystemRestfulInteraction> src) throws FHIRException { 781 if (src == null || src.isEmpty()) 782 return null; 783 org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Conformance.SystemRestfulInteraction> tgt = new org.hl7.fhir.dstu2016may.model.Enumeration<>(new org.hl7.fhir.dstu2016may.model.Conformance.SystemRestfulInteractionEnumFactory()); 784 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyElement(src, tgt); 785 switch (src.getValue()) { 786 case TRANSACTION: 787 tgt.setValue(org.hl7.fhir.dstu2016may.model.Conformance.SystemRestfulInteraction.TRANSACTION); 788 break; 789 case SEARCHSYSTEM: 790 tgt.setValue(org.hl7.fhir.dstu2016may.model.Conformance.SystemRestfulInteraction.SEARCHSYSTEM); 791 break; 792 case HISTORYSYSTEM: 793 tgt.setValue(org.hl7.fhir.dstu2016may.model.Conformance.SystemRestfulInteraction.HISTORYSYSTEM); 794 break; 795 default: 796 tgt.setValue(org.hl7.fhir.dstu2016may.model.Conformance.SystemRestfulInteraction.NULL); 797 break; 798 } 799 return tgt; 800 } 801 802 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.CapabilityStatement.SystemRestfulInteraction> convertSystemRestfulInteraction(org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Conformance.SystemRestfulInteraction> src) throws FHIRException { 803 if (src == null || src.isEmpty()) 804 return null; 805 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.CapabilityStatement.SystemRestfulInteraction> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.CapabilityStatement.SystemRestfulInteractionEnumFactory()); 806 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyElement(src, tgt); 807 switch (src.getValue()) { 808 case TRANSACTION: 809 tgt.setValue(org.hl7.fhir.r4.model.CapabilityStatement.SystemRestfulInteraction.TRANSACTION); 810 break; 811 case SEARCHSYSTEM: 812 tgt.setValue(org.hl7.fhir.r4.model.CapabilityStatement.SystemRestfulInteraction.SEARCHSYSTEM); 813 break; 814 case HISTORYSYSTEM: 815 tgt.setValue(org.hl7.fhir.r4.model.CapabilityStatement.SystemRestfulInteraction.HISTORYSYSTEM); 816 break; 817 default: 818 tgt.setValue(org.hl7.fhir.r4.model.CapabilityStatement.SystemRestfulInteraction.NULL); 819 break; 820 } 821 return tgt; 822 } 823 824 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.CapabilityStatement.TypeRestfulInteraction> convertTypeRestfulInteraction(org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Conformance.TypeRestfulInteraction> src) throws FHIRException { 825 if (src == null || src.isEmpty()) 826 return null; 827 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.CapabilityStatement.TypeRestfulInteraction> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.CapabilityStatement.TypeRestfulInteractionEnumFactory()); 828 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyElement(src, tgt); 829 switch (src.getValue()) { 830 case READ: 831 tgt.setValue(org.hl7.fhir.r4.model.CapabilityStatement.TypeRestfulInteraction.READ); 832 break; 833 case VREAD: 834 tgt.setValue(org.hl7.fhir.r4.model.CapabilityStatement.TypeRestfulInteraction.VREAD); 835 break; 836 case UPDATE: 837 tgt.setValue(org.hl7.fhir.r4.model.CapabilityStatement.TypeRestfulInteraction.UPDATE); 838 break; 839 case DELETE: 840 tgt.setValue(org.hl7.fhir.r4.model.CapabilityStatement.TypeRestfulInteraction.DELETE); 841 break; 842 case HISTORYINSTANCE: 843 tgt.setValue(org.hl7.fhir.r4.model.CapabilityStatement.TypeRestfulInteraction.HISTORYINSTANCE); 844 break; 845 case HISTORYTYPE: 846 tgt.setValue(org.hl7.fhir.r4.model.CapabilityStatement.TypeRestfulInteraction.HISTORYTYPE); 847 break; 848 case CREATE: 849 tgt.setValue(org.hl7.fhir.r4.model.CapabilityStatement.TypeRestfulInteraction.CREATE); 850 break; 851 case SEARCHTYPE: 852 tgt.setValue(org.hl7.fhir.r4.model.CapabilityStatement.TypeRestfulInteraction.SEARCHTYPE); 853 break; 854 default: 855 tgt.setValue(org.hl7.fhir.r4.model.CapabilityStatement.TypeRestfulInteraction.NULL); 856 break; 857 } 858 return tgt; 859 } 860 861 static public org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Conformance.TypeRestfulInteraction> convertTypeRestfulInteraction(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.CapabilityStatement.TypeRestfulInteraction> src) throws FHIRException { 862 if (src == null || src.isEmpty()) 863 return null; 864 org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Conformance.TypeRestfulInteraction> tgt = new org.hl7.fhir.dstu2016may.model.Enumeration<>(new org.hl7.fhir.dstu2016may.model.Conformance.TypeRestfulInteractionEnumFactory()); 865 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyElement(src, tgt); 866 switch (src.getValue()) { 867 case READ: 868 tgt.setValue(org.hl7.fhir.dstu2016may.model.Conformance.TypeRestfulInteraction.READ); 869 break; 870 case VREAD: 871 tgt.setValue(org.hl7.fhir.dstu2016may.model.Conformance.TypeRestfulInteraction.VREAD); 872 break; 873 case UPDATE: 874 tgt.setValue(org.hl7.fhir.dstu2016may.model.Conformance.TypeRestfulInteraction.UPDATE); 875 break; 876 case DELETE: 877 tgt.setValue(org.hl7.fhir.dstu2016may.model.Conformance.TypeRestfulInteraction.DELETE); 878 break; 879 case HISTORYINSTANCE: 880 tgt.setValue(org.hl7.fhir.dstu2016may.model.Conformance.TypeRestfulInteraction.HISTORYINSTANCE); 881 break; 882 case HISTORYTYPE: 883 tgt.setValue(org.hl7.fhir.dstu2016may.model.Conformance.TypeRestfulInteraction.HISTORYTYPE); 884 break; 885 case CREATE: 886 tgt.setValue(org.hl7.fhir.dstu2016may.model.Conformance.TypeRestfulInteraction.CREATE); 887 break; 888 case SEARCHTYPE: 889 tgt.setValue(org.hl7.fhir.dstu2016may.model.Conformance.TypeRestfulInteraction.SEARCHTYPE); 890 break; 891 default: 892 tgt.setValue(org.hl7.fhir.dstu2016may.model.Conformance.TypeRestfulInteraction.NULL); 893 break; 894 } 895 return tgt; 896 } 897 898 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.CapabilityStatement.EventCapabilityMode> convertConformanceEventMode(org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Conformance.ConformanceEventMode> src) throws FHIRException { 899 if (src == null || src.isEmpty()) return null; 900 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.CapabilityStatement.EventCapabilityMode> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.CapabilityStatement.EventCapabilityModeEnumFactory()); 901 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyElement(src, tgt); 902 if (src.getValue() == null) { 903 tgt.setValue(org.hl7.fhir.r4.model.CapabilityStatement.EventCapabilityMode.NULL); 904 } else { 905 switch (src.getValue()) { 906 case SENDER: 907 tgt.setValue(org.hl7.fhir.r4.model.CapabilityStatement.EventCapabilityMode.SENDER); 908 break; 909 case RECEIVER: 910 tgt.setValue(org.hl7.fhir.r4.model.CapabilityStatement.EventCapabilityMode.RECEIVER); 911 break; 912 default: 913 tgt.setValue(org.hl7.fhir.r4.model.CapabilityStatement.EventCapabilityMode.NULL); 914 break; 915 } 916 } 917 return tgt; 918 } 919 920 static public org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Conformance.ConformanceEventMode> convertConformanceEventMode(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.CapabilityStatement.EventCapabilityMode> src) throws FHIRException { 921 if (src == null || src.isEmpty()) return null; 922 org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Conformance.ConformanceEventMode> tgt = new org.hl7.fhir.dstu2016may.model.Enumeration<>(new org.hl7.fhir.dstu2016may.model.Conformance.ConformanceEventModeEnumFactory()); 923 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyElement(src, tgt); 924 if (src.getValue() == null) { 925 tgt.setValue(org.hl7.fhir.dstu2016may.model.Conformance.ConformanceEventMode.NULL); 926 } else { 927 switch (src.getValue()) { 928 case SENDER: 929 tgt.setValue(org.hl7.fhir.dstu2016may.model.Conformance.ConformanceEventMode.SENDER); 930 break; 931 case RECEIVER: 932 tgt.setValue(org.hl7.fhir.dstu2016may.model.Conformance.ConformanceEventMode.RECEIVER); 933 break; 934 default: 935 tgt.setValue(org.hl7.fhir.dstu2016may.model.Conformance.ConformanceEventMode.NULL); 936 break; 937 } 938 } 939 return tgt; 940 } 941}