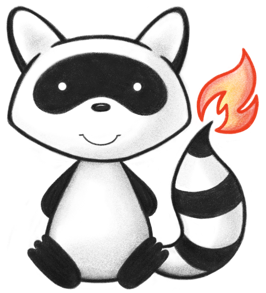
001package org.hl7.fhir.convertors.conv14_40.resources14_40; 002 003import org.hl7.fhir.convertors.context.ConversionContext14_40; 004import org.hl7.fhir.convertors.conv14_40.datatypes14_40.complextypes14_40.Signature14_40; 005import org.hl7.fhir.convertors.conv14_40.datatypes14_40.primitivetypes14_40.Decimal14_40; 006import org.hl7.fhir.convertors.conv14_40.datatypes14_40.primitivetypes14_40.Instant14_40; 007import org.hl7.fhir.convertors.conv14_40.datatypes14_40.primitivetypes14_40.String14_40; 008import org.hl7.fhir.convertors.conv14_40.datatypes14_40.primitivetypes14_40.UnsignedInt14_40; 009import org.hl7.fhir.convertors.conv14_40.datatypes14_40.primitivetypes14_40.Uri14_40; 010import org.hl7.fhir.exceptions.FHIRException; 011 012public class Bundle14_40 { 013 014 public static org.hl7.fhir.r4.model.Bundle convertBundle(org.hl7.fhir.dstu2016may.model.Bundle src) throws FHIRException { 015 if (src == null || src.isEmpty()) 016 return null; 017 org.hl7.fhir.r4.model.Bundle tgt = new org.hl7.fhir.r4.model.Bundle(); 018 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyResource(src, tgt); 019 if (src.hasType()) 020 tgt.setTypeElement(convertBundleType(src.getTypeElement())); 021 if (src.hasTotal()) 022 tgt.setTotalElement(UnsignedInt14_40.convertUnsignedInt(src.getTotalElement())); 023 for (org.hl7.fhir.dstu2016may.model.Bundle.BundleLinkComponent t : src.getLink()) 024 tgt.addLink(convertBundleLinkComponent(t)); 025 for (org.hl7.fhir.dstu2016may.model.Bundle.BundleEntryComponent t : src.getEntry()) 026 tgt.addEntry(convertBundleEntryComponent(t)); 027 if (src.hasSignature()) 028 tgt.setSignature(Signature14_40.convertSignature(src.getSignature())); 029 return tgt; 030 } 031 032 public static org.hl7.fhir.dstu2016may.model.Bundle convertBundle(org.hl7.fhir.r4.model.Bundle src) throws FHIRException { 033 if (src == null || src.isEmpty()) 034 return null; 035 org.hl7.fhir.dstu2016may.model.Bundle tgt = new org.hl7.fhir.dstu2016may.model.Bundle(); 036 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyResource(src, tgt); 037 if (src.hasType()) 038 tgt.setTypeElement(convertBundleType(src.getTypeElement())); 039 if (src.hasTotal()) 040 tgt.setTotalElement(UnsignedInt14_40.convertUnsignedInt(src.getTotalElement())); 041 for (org.hl7.fhir.r4.model.Bundle.BundleLinkComponent t : src.getLink()) tgt.addLink(convertBundleLinkComponent(t)); 042 for (org.hl7.fhir.r4.model.Bundle.BundleEntryComponent t : src.getEntry()) 043 tgt.addEntry(convertBundleEntryComponent(t)); 044 if (src.hasSignature()) 045 tgt.setSignature(Signature14_40.convertSignature(src.getSignature())); 046 return tgt; 047 } 048 049 public static org.hl7.fhir.r4.model.Bundle.BundleEntryComponent convertBundleEntryComponent(org.hl7.fhir.dstu2016may.model.Bundle.BundleEntryComponent src) throws FHIRException { 050 if (src == null || src.isEmpty()) 051 return null; 052 org.hl7.fhir.r4.model.Bundle.BundleEntryComponent tgt = new org.hl7.fhir.r4.model.Bundle.BundleEntryComponent(); 053 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyBackboneElement(src,tgt); 054 for (org.hl7.fhir.dstu2016may.model.Bundle.BundleLinkComponent t : src.getLink()) 055 tgt.addLink(convertBundleLinkComponent(t)); 056 if (src.hasFullUrl()) 057 tgt.setFullUrlElement(Uri14_40.convertUri(src.getFullUrlElement())); 058 if (src.hasResource()) 059 tgt.setResource(ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().convertResource(src.getResource())); 060 if (src.hasSearch()) 061 tgt.setSearch(convertBundleEntrySearchComponent(src.getSearch())); 062 if (src.hasRequest()) 063 tgt.setRequest(convertBundleEntryRequestComponent(src.getRequest())); 064 if (src.hasResponse()) 065 tgt.setResponse(convertBundleEntryResponseComponent(src.getResponse())); 066 return tgt; 067 } 068 069 public static org.hl7.fhir.dstu2016may.model.Bundle.BundleEntryComponent convertBundleEntryComponent(org.hl7.fhir.r4.model.Bundle.BundleEntryComponent src) throws FHIRException { 070 if (src == null || src.isEmpty()) 071 return null; 072 org.hl7.fhir.dstu2016may.model.Bundle.BundleEntryComponent tgt = new org.hl7.fhir.dstu2016may.model.Bundle.BundleEntryComponent(); 073 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyBackboneElement(src,tgt); 074 for (org.hl7.fhir.r4.model.Bundle.BundleLinkComponent t : src.getLink()) tgt.addLink(convertBundleLinkComponent(t)); 075 if (src.hasFullUrl()) 076 tgt.setFullUrlElement(Uri14_40.convertUri(src.getFullUrlElement())); 077 if (src.hasResource()) 078 tgt.setResource(ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().convertResource(src.getResource())); 079 if (src.hasSearch()) 080 tgt.setSearch(convertBundleEntrySearchComponent(src.getSearch())); 081 if (src.hasRequest()) 082 tgt.setRequest(convertBundleEntryRequestComponent(src.getRequest())); 083 if (src.hasResponse()) 084 tgt.setResponse(convertBundleEntryResponseComponent(src.getResponse())); 085 return tgt; 086 } 087 088 public static org.hl7.fhir.r4.model.Bundle.BundleEntryRequestComponent convertBundleEntryRequestComponent(org.hl7.fhir.dstu2016may.model.Bundle.BundleEntryRequestComponent src) throws FHIRException { 089 if (src == null || src.isEmpty()) 090 return null; 091 org.hl7.fhir.r4.model.Bundle.BundleEntryRequestComponent tgt = new org.hl7.fhir.r4.model.Bundle.BundleEntryRequestComponent(); 092 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyBackboneElement(src,tgt); 093 if (src.hasMethod()) 094 tgt.setMethodElement(convertHTTPVerb(src.getMethodElement())); 095 if (src.hasUrlElement()) 096 tgt.setUrlElement(Uri14_40.convertUri(src.getUrlElement())); 097 if (src.hasIfNoneMatch()) 098 tgt.setIfNoneMatchElement(String14_40.convertString(src.getIfNoneMatchElement())); 099 if (src.hasIfModifiedSince()) 100 tgt.setIfModifiedSinceElement(Instant14_40.convertInstant(src.getIfModifiedSinceElement())); 101 if (src.hasIfMatch()) 102 tgt.setIfMatchElement(String14_40.convertString(src.getIfMatchElement())); 103 if (src.hasIfNoneExist()) 104 tgt.setIfNoneExistElement(String14_40.convertString(src.getIfNoneExistElement())); 105 return tgt; 106 } 107 108 public static org.hl7.fhir.dstu2016may.model.Bundle.BundleEntryRequestComponent convertBundleEntryRequestComponent(org.hl7.fhir.r4.model.Bundle.BundleEntryRequestComponent src) throws FHIRException { 109 if (src == null || src.isEmpty()) 110 return null; 111 org.hl7.fhir.dstu2016may.model.Bundle.BundleEntryRequestComponent tgt = new org.hl7.fhir.dstu2016may.model.Bundle.BundleEntryRequestComponent(); 112 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyBackboneElement(src,tgt); 113 if (src.hasMethod()) 114 tgt.setMethodElement(convertHTTPVerb(src.getMethodElement())); 115 if (src.hasUrlElement()) 116 tgt.setUrlElement(Uri14_40.convertUri(src.getUrlElement())); 117 if (src.hasIfNoneMatch()) 118 tgt.setIfNoneMatchElement(String14_40.convertString(src.getIfNoneMatchElement())); 119 if (src.hasIfModifiedSince()) 120 tgt.setIfModifiedSinceElement(Instant14_40.convertInstant(src.getIfModifiedSinceElement())); 121 if (src.hasIfMatch()) 122 tgt.setIfMatchElement(String14_40.convertString(src.getIfMatchElement())); 123 if (src.hasIfNoneExist()) 124 tgt.setIfNoneExistElement(String14_40.convertString(src.getIfNoneExistElement())); 125 return tgt; 126 } 127 128 public static org.hl7.fhir.r4.model.Bundle.BundleEntryResponseComponent convertBundleEntryResponseComponent(org.hl7.fhir.dstu2016may.model.Bundle.BundleEntryResponseComponent src) throws FHIRException { 129 if (src == null || src.isEmpty()) 130 return null; 131 org.hl7.fhir.r4.model.Bundle.BundleEntryResponseComponent tgt = new org.hl7.fhir.r4.model.Bundle.BundleEntryResponseComponent(); 132 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyBackboneElement(src,tgt); 133 if (src.hasStatusElement()) 134 tgt.setStatusElement(String14_40.convertString(src.getStatusElement())); 135 if (src.hasLocation()) 136 tgt.setLocationElement(Uri14_40.convertUri(src.getLocationElement())); 137 if (src.hasEtag()) 138 tgt.setEtagElement(String14_40.convertString(src.getEtagElement())); 139 if (src.hasLastModified()) 140 tgt.setLastModifiedElement(Instant14_40.convertInstant(src.getLastModifiedElement())); 141 return tgt; 142 } 143 144 public static org.hl7.fhir.dstu2016may.model.Bundle.BundleEntryResponseComponent convertBundleEntryResponseComponent(org.hl7.fhir.r4.model.Bundle.BundleEntryResponseComponent src) throws FHIRException { 145 if (src == null || src.isEmpty()) 146 return null; 147 org.hl7.fhir.dstu2016may.model.Bundle.BundleEntryResponseComponent tgt = new org.hl7.fhir.dstu2016may.model.Bundle.BundleEntryResponseComponent(); 148 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyBackboneElement(src,tgt); 149 if (src.hasStatusElement()) 150 tgt.setStatusElement(String14_40.convertString(src.getStatusElement())); 151 if (src.hasLocation()) 152 tgt.setLocationElement(Uri14_40.convertUri(src.getLocationElement())); 153 if (src.hasEtag()) 154 tgt.setEtagElement(String14_40.convertString(src.getEtagElement())); 155 if (src.hasLastModified()) 156 tgt.setLastModifiedElement(Instant14_40.convertInstant(src.getLastModifiedElement())); 157 return tgt; 158 } 159 160 public static org.hl7.fhir.dstu2016may.model.Bundle.BundleEntrySearchComponent convertBundleEntrySearchComponent(org.hl7.fhir.r4.model.Bundle.BundleEntrySearchComponent src) throws FHIRException { 161 if (src == null || src.isEmpty()) 162 return null; 163 org.hl7.fhir.dstu2016may.model.Bundle.BundleEntrySearchComponent tgt = new org.hl7.fhir.dstu2016may.model.Bundle.BundleEntrySearchComponent(); 164 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyBackboneElement(src,tgt); 165 if (src.hasMode()) 166 tgt.setModeElement(convertSearchEntryMode(src.getModeElement())); 167 if (src.hasScore()) 168 tgt.setScoreElement(Decimal14_40.convertDecimal(src.getScoreElement())); 169 return tgt; 170 } 171 172 public static org.hl7.fhir.r4.model.Bundle.BundleEntrySearchComponent convertBundleEntrySearchComponent(org.hl7.fhir.dstu2016may.model.Bundle.BundleEntrySearchComponent src) throws FHIRException { 173 if (src == null || src.isEmpty()) 174 return null; 175 org.hl7.fhir.r4.model.Bundle.BundleEntrySearchComponent tgt = new org.hl7.fhir.r4.model.Bundle.BundleEntrySearchComponent(); 176 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyBackboneElement(src,tgt); 177 if (src.hasMode()) 178 tgt.setModeElement(convertSearchEntryMode(src.getModeElement())); 179 if (src.hasScore()) 180 tgt.setScoreElement(Decimal14_40.convertDecimal(src.getScoreElement())); 181 return tgt; 182 } 183 184 public static org.hl7.fhir.dstu2016may.model.Bundle.BundleLinkComponent convertBundleLinkComponent(org.hl7.fhir.r4.model.Bundle.BundleLinkComponent src) throws FHIRException { 185 if (src == null || src.isEmpty()) 186 return null; 187 org.hl7.fhir.dstu2016may.model.Bundle.BundleLinkComponent tgt = new org.hl7.fhir.dstu2016may.model.Bundle.BundleLinkComponent(); 188 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyBackboneElement(src,tgt); 189 if (src.hasRelationElement()) 190 tgt.setRelationElement(String14_40.convertString(src.getRelationElement())); 191 if (src.hasUrlElement()) 192 tgt.setUrlElement(Uri14_40.convertUri(src.getUrlElement())); 193 return tgt; 194 } 195 196 public static org.hl7.fhir.r4.model.Bundle.BundleLinkComponent convertBundleLinkComponent(org.hl7.fhir.dstu2016may.model.Bundle.BundleLinkComponent src) throws FHIRException { 197 if (src == null || src.isEmpty()) 198 return null; 199 org.hl7.fhir.r4.model.Bundle.BundleLinkComponent tgt = new org.hl7.fhir.r4.model.Bundle.BundleLinkComponent(); 200 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyBackboneElement(src,tgt); 201 if (src.hasRelationElement()) 202 tgt.setRelationElement(String14_40.convertString(src.getRelationElement())); 203 if (src.hasUrlElement()) 204 tgt.setUrlElement(Uri14_40.convertUri(src.getUrlElement())); 205 return tgt; 206 } 207 208 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Bundle.BundleType> convertBundleType(org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Bundle.BundleType> src) throws FHIRException { 209 if (src == null || src.isEmpty()) 210 return null; 211 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Bundle.BundleType> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.Bundle.BundleTypeEnumFactory()); 212 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyElement(src, tgt); 213 switch (src.getValue()) { 214 case DOCUMENT: 215 tgt.setValue(org.hl7.fhir.r4.model.Bundle.BundleType.DOCUMENT); 216 break; 217 case MESSAGE: 218 tgt.setValue(org.hl7.fhir.r4.model.Bundle.BundleType.MESSAGE); 219 break; 220 case TRANSACTION: 221 tgt.setValue(org.hl7.fhir.r4.model.Bundle.BundleType.TRANSACTION); 222 break; 223 case TRANSACTIONRESPONSE: 224 tgt.setValue(org.hl7.fhir.r4.model.Bundle.BundleType.TRANSACTIONRESPONSE); 225 break; 226 case BATCH: 227 tgt.setValue(org.hl7.fhir.r4.model.Bundle.BundleType.BATCH); 228 break; 229 case BATCHRESPONSE: 230 tgt.setValue(org.hl7.fhir.r4.model.Bundle.BundleType.BATCHRESPONSE); 231 break; 232 case HISTORY: 233 tgt.setValue(org.hl7.fhir.r4.model.Bundle.BundleType.HISTORY); 234 break; 235 case SEARCHSET: 236 tgt.setValue(org.hl7.fhir.r4.model.Bundle.BundleType.SEARCHSET); 237 break; 238 case COLLECTION: 239 tgt.setValue(org.hl7.fhir.r4.model.Bundle.BundleType.COLLECTION); 240 break; 241 default: 242 tgt.setValue(org.hl7.fhir.r4.model.Bundle.BundleType.NULL); 243 break; 244 } 245 return tgt; 246 } 247 248 static public org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Bundle.BundleType> convertBundleType(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Bundle.BundleType> src) throws FHIRException { 249 if (src == null || src.isEmpty()) 250 return null; 251 org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Bundle.BundleType> tgt = new org.hl7.fhir.dstu2016may.model.Enumeration<>(new org.hl7.fhir.dstu2016may.model.Bundle.BundleTypeEnumFactory()); 252 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyElement(src, tgt); 253 switch (src.getValue()) { 254 case DOCUMENT: 255 tgt.setValue(org.hl7.fhir.dstu2016may.model.Bundle.BundleType.DOCUMENT); 256 break; 257 case MESSAGE: 258 tgt.setValue(org.hl7.fhir.dstu2016may.model.Bundle.BundleType.MESSAGE); 259 break; 260 case TRANSACTION: 261 tgt.setValue(org.hl7.fhir.dstu2016may.model.Bundle.BundleType.TRANSACTION); 262 break; 263 case TRANSACTIONRESPONSE: 264 tgt.setValue(org.hl7.fhir.dstu2016may.model.Bundle.BundleType.TRANSACTIONRESPONSE); 265 break; 266 case BATCH: 267 tgt.setValue(org.hl7.fhir.dstu2016may.model.Bundle.BundleType.BATCH); 268 break; 269 case BATCHRESPONSE: 270 tgt.setValue(org.hl7.fhir.dstu2016may.model.Bundle.BundleType.BATCHRESPONSE); 271 break; 272 case HISTORY: 273 tgt.setValue(org.hl7.fhir.dstu2016may.model.Bundle.BundleType.HISTORY); 274 break; 275 case SEARCHSET: 276 tgt.setValue(org.hl7.fhir.dstu2016may.model.Bundle.BundleType.SEARCHSET); 277 break; 278 case COLLECTION: 279 tgt.setValue(org.hl7.fhir.dstu2016may.model.Bundle.BundleType.COLLECTION); 280 break; 281 default: 282 tgt.setValue(org.hl7.fhir.dstu2016may.model.Bundle.BundleType.NULL); 283 break; 284 } 285 return tgt; 286 } 287 288 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Bundle.HTTPVerb> convertHTTPVerb(org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Bundle.HTTPVerb> src) throws FHIRException { 289 if (src == null || src.isEmpty()) 290 return null; 291 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Bundle.HTTPVerb> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.Bundle.HTTPVerbEnumFactory()); 292 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyElement(src, tgt); 293 switch (src.getValue()) { 294 case GET: 295 tgt.setValue(org.hl7.fhir.r4.model.Bundle.HTTPVerb.GET); 296 break; 297 case POST: 298 tgt.setValue(org.hl7.fhir.r4.model.Bundle.HTTPVerb.POST); 299 break; 300 case PUT: 301 tgt.setValue(org.hl7.fhir.r4.model.Bundle.HTTPVerb.PUT); 302 break; 303 case DELETE: 304 tgt.setValue(org.hl7.fhir.r4.model.Bundle.HTTPVerb.DELETE); 305 break; 306 default: 307 tgt.setValue(org.hl7.fhir.r4.model.Bundle.HTTPVerb.NULL); 308 break; 309 } 310 return tgt; 311 } 312 313 static public org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Bundle.HTTPVerb> convertHTTPVerb(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Bundle.HTTPVerb> src) throws FHIRException { 314 if (src == null || src.isEmpty()) 315 return null; 316 org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Bundle.HTTPVerb> tgt = new org.hl7.fhir.dstu2016may.model.Enumeration<>(new org.hl7.fhir.dstu2016may.model.Bundle.HTTPVerbEnumFactory()); 317 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyElement(src, tgt); 318 switch (src.getValue()) { 319 case GET: 320 tgt.setValue(org.hl7.fhir.dstu2016may.model.Bundle.HTTPVerb.GET); 321 break; 322 case POST: 323 tgt.setValue(org.hl7.fhir.dstu2016may.model.Bundle.HTTPVerb.POST); 324 break; 325 case PUT: 326 tgt.setValue(org.hl7.fhir.dstu2016may.model.Bundle.HTTPVerb.PUT); 327 break; 328 case DELETE: 329 tgt.setValue(org.hl7.fhir.dstu2016may.model.Bundle.HTTPVerb.DELETE); 330 break; 331 default: 332 tgt.setValue(org.hl7.fhir.dstu2016may.model.Bundle.HTTPVerb.NULL); 333 break; 334 } 335 return tgt; 336 } 337 338 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Bundle.SearchEntryMode> convertSearchEntryMode(org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Bundle.SearchEntryMode> src) throws FHIRException { 339 if (src == null || src.isEmpty()) 340 return null; 341 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Bundle.SearchEntryMode> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.Bundle.SearchEntryModeEnumFactory()); 342 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyElement(src, tgt); 343 switch (src.getValue()) { 344 case MATCH: 345 tgt.setValue(org.hl7.fhir.r4.model.Bundle.SearchEntryMode.MATCH); 346 break; 347 case INCLUDE: 348 tgt.setValue(org.hl7.fhir.r4.model.Bundle.SearchEntryMode.INCLUDE); 349 break; 350 case OUTCOME: 351 tgt.setValue(org.hl7.fhir.r4.model.Bundle.SearchEntryMode.OUTCOME); 352 break; 353 default: 354 tgt.setValue(org.hl7.fhir.r4.model.Bundle.SearchEntryMode.NULL); 355 break; 356 } 357 return tgt; 358 } 359 360 static public org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Bundle.SearchEntryMode> convertSearchEntryMode(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Bundle.SearchEntryMode> src) throws FHIRException { 361 if (src == null || src.isEmpty()) 362 return null; 363 org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Bundle.SearchEntryMode> tgt = new org.hl7.fhir.dstu2016may.model.Enumeration<>(new org.hl7.fhir.dstu2016may.model.Bundle.SearchEntryModeEnumFactory()); 364 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyElement(src, tgt); 365 switch (src.getValue()) { 366 case MATCH: 367 tgt.setValue(org.hl7.fhir.dstu2016may.model.Bundle.SearchEntryMode.MATCH); 368 break; 369 case INCLUDE: 370 tgt.setValue(org.hl7.fhir.dstu2016may.model.Bundle.SearchEntryMode.INCLUDE); 371 break; 372 case OUTCOME: 373 tgt.setValue(org.hl7.fhir.dstu2016may.model.Bundle.SearchEntryMode.OUTCOME); 374 break; 375 default: 376 tgt.setValue(org.hl7.fhir.dstu2016may.model.Bundle.SearchEntryMode.NULL); 377 break; 378 } 379 return tgt; 380 } 381}