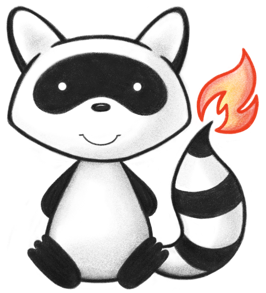
001/* 002 * #%L 003 * HAPI FHIR - Client Framework 004 * %% 005 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.client.interceptor; 021 022import ca.uhn.fhir.rest.client.api.IClientInterceptor; 023import ca.uhn.fhir.rest.client.api.IHttpRequest; 024import ca.uhn.fhir.rest.client.api.IHttpResponse; 025import org.apache.commons.lang3.Validate; 026 027import java.io.IOException; 028 029import static org.apache.commons.lang3.StringUtils.isNotBlank; 030 031/** 032 * This interceptor adds an arbitrary header to requests made by this client. Both the 033 * header name and the header value are specified by the calling code. 034 * 035 * @see AdditionalRequestHeadersInterceptor for a more advanced version of this interceptor which can add multiple headers 036 */ 037public class SimpleRequestHeaderInterceptor implements IClientInterceptor { 038 039 private String myHeaderName; 040 private String myHeaderValue; 041 042 /** 043 * Constructor 044 */ 045 public SimpleRequestHeaderInterceptor() { 046 this(null, null); 047 } 048 049 /** 050 * Constructor 051 * 052 * @param theHeaderName The header name, e.g. "<code>Authorization</code>" 053 * @param theHeaderValue The header value, e.g. "<code>Bearer 09uer90uw9yh</code>" 054 */ 055 public SimpleRequestHeaderInterceptor(String theHeaderName, String theHeaderValue) { 056 super(); 057 myHeaderName = theHeaderName; 058 myHeaderValue = theHeaderValue; 059 } 060 061 /** 062 * Constructor which takes a complete header including name and value 063 * 064 * @param theCompleteHeader The complete header, e.g. "<code>Authorization: Bearer af09ufe90efh</code>". Must not be null or empty. 065 */ 066 public SimpleRequestHeaderInterceptor(String theCompleteHeader) { 067 Validate.notBlank(theCompleteHeader, "theCompleteHeader must not be null"); 068 069 int colonIdx = theCompleteHeader.indexOf(':'); 070 if (colonIdx != -1) { 071 setHeaderName(theCompleteHeader.substring(0, colonIdx).trim()); 072 setHeaderValue(theCompleteHeader 073 .substring(colonIdx + 1, theCompleteHeader.length()) 074 .trim()); 075 } else { 076 setHeaderName(theCompleteHeader.trim()); 077 setHeaderValue(null); 078 } 079 } 080 081 public String getHeaderName() { 082 return myHeaderName; 083 } 084 085 public String getHeaderValue() { 086 return myHeaderValue; 087 } 088 089 @Override 090 public void interceptRequest(IHttpRequest theRequest) { 091 if (isNotBlank(getHeaderName())) { 092 theRequest.addHeader(getHeaderName(), getHeaderValue()); 093 } 094 } 095 096 @Override 097 public void interceptResponse(IHttpResponse theResponse) throws IOException { 098 // nothing 099 } 100 101 public void setHeaderName(String theHeaderName) { 102 myHeaderName = theHeaderName; 103 } 104 105 public void setHeaderValue(String theHeaderValue) { 106 myHeaderValue = theHeaderValue; 107 } 108}