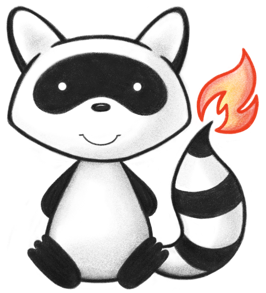
001/* 002 * #%L 003 * HAPI FHIR - Client Framework 004 * %% 005 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.client.apache; 021 022import ca.uhn.fhir.rest.api.Constants; 023import ca.uhn.fhir.rest.client.api.IClientInterceptor; 024import ca.uhn.fhir.rest.client.api.IHttpRequest; 025import ca.uhn.fhir.rest.client.api.IHttpResponse; 026import org.apache.http.Header; 027import org.apache.http.HttpEntityEnclosingRequest; 028import org.apache.http.client.methods.HttpRequestBase; 029import org.apache.http.entity.ByteArrayEntity; 030 031import java.io.ByteArrayOutputStream; 032import java.io.IOException; 033import java.util.zip.GZIPOutputStream; 034 035/** 036 * Client interceptor which GZip compresses outgoing (POST/PUT) contents being uploaded 037 * from the client to the server. This can improve performance by reducing network 038 * load time. 039 */ 040public class GZipContentInterceptor implements IClientInterceptor { 041 private static final org.slf4j.Logger ourLog = org.slf4j.LoggerFactory.getLogger(GZipContentInterceptor.class); 042 043 @Override 044 public void interceptRequest(IHttpRequest theRequestInterface) { 045 HttpRequestBase theRequest = ((ApacheHttpRequest) theRequestInterface).getApacheRequest(); 046 if (theRequest instanceof HttpEntityEnclosingRequest) { 047 Header[] encodingHeaders = theRequest.getHeaders(Constants.HEADER_CONTENT_ENCODING); 048 if (encodingHeaders == null || encodingHeaders.length == 0) { 049 HttpEntityEnclosingRequest req = (HttpEntityEnclosingRequest) theRequest; 050 051 ByteArrayOutputStream bos = new ByteArrayOutputStream(); 052 GZIPOutputStream gos; 053 try { 054 gos = new GZIPOutputStream(bos); 055 req.getEntity().writeTo(gos); 056 gos.finish(); 057 } catch (IOException e) { 058 ourLog.warn("Failed to GZip outgoing content", e); 059 return; 060 } 061 062 byte[] byteArray = bos.toByteArray(); 063 ByteArrayEntity newEntity = new ByteArrayEntity(byteArray); 064 req.setEntity(newEntity); 065 req.addHeader(Constants.HEADER_CONTENT_ENCODING, "gzip"); 066 } 067 } 068 } 069 070 @Override 071 public void interceptResponse(IHttpResponse theResponse) throws IOException { 072 // nothing 073 } 074}